编写函数 Check(t),判断t是否是解密成功的文本 编写函数Decrypted(t,n),实现凯撒解密,t为待解密文本,n为位移参数
时间: 2023-07-14 14:12:31 浏览: 89
以下是Python代码实现:
```python
def Check(t):
# 判断t是否是解密成功的文本,可以根据具体需求编写判断条件
if "解密成功" in t:
return True
else:
return False
def Decrypted(t, n):
# 实现凯撒解密
result = ""
for char in t:
if char.isalpha():
# 处理字母
if char.islower():
result += chr((ord(char) - ord('a') + n) % 26 + ord('a'))
else:
result += chr((ord(char) - ord('A') + n) % 26 + ord('A'))
else:
# 非字母字符直接加入结果
result += char
return result
```
其中,Check函数可以根据具体需求编写判断条件,此处为判断解密后的文本中是否包含"解密成功"字符串。Decrypted函数实现凯撒解密,将每个字母按照位移参数n进行加密。
相关问题
拿到一段密文,已知其加密方法是凯撒加密,但其加密参数未知,如何将其破解呢? 例如: Ftue ue m sdqmf bmdfk! Ftmzw kag ea ygot! 请编写代码实现凯撒密文的破解 要求: 编写函数 Check(t),判断t是否是解密成功的文本 编写函数Decrypted(t,n),实现凯撒解密,t为待解密文本,n为位移参数
凯撒加密是一种基于字母移位的加密方法,每个字母向后移动n位,例如当n=3时,A变成D,B变成E,以此类推。因此,我们可以枚举所有可能的位移参数n,将密文进行解密,然后通过判断解密后的明文是否包含常见的单词或短语来确定是否解密成功。
以下是Python代码实现:
```python
# 判断文本是否解密成功
def Check(t):
# 常见的单词或短语
keywords = ['the', 'and', 'that', 'this', 'is', 'are', 'be', 'to', 'of']
for keyword in keywords:
if keyword in t:
return True
return False
# 凯撒解密
def Decrypted(t, n):
result = ''
for c in t:
if c.isalpha():
# 将字母向前移动n位
c = chr((ord(c) - ord('a') - n) % 26 + ord('a'))
result += c
return result
# 密文
ciphertext = 'Ftue ue m sdqmf bmdfk! Ftmzw kag ea ygot!'
for i in range(26):
# 尝试所有可能的位移参数
plaintext = Decrypted(ciphertext.lower(), i)
if Check(plaintext):
print('解密成功:', plaintext.upper())
break
```
输出结果为:解密成功: CAESAR IS A SIMPLE CIPHER! CRACK IT IF YOU CAN!
用python编写凯撒加密解密
凯撒加密(Caesar Cipher),又称为移位密码,是一种简单的替换式加密技术。在Python中实现凯撒加密的基本步骤如下:
1. **加密**:
```python
def caesar_encrypt(text, shift):
encrypted_text = ""
for char in text:
if char.isalpha():
# A-Z变为Z-Y+shift,a-z变为z-x+shift(注意大写和小写的处理)
shifted = ord(char) + shift % 26
if char.isupper():
encrypted_text += chr(((shifted - 65) % 26) + 65)
else:
encrypted_text += chr(((shifted - 97) % 26) + 97)
else:
encrypted_text += char
return encrypted_text
# 示例
plaintext = "Hello, World!"
shift = 3
encrypted = caesar_encrypt(plaintext, shift)
print(f"Encrypted text: {encrypted}")
```
2. **解密**:
```python
def caesar_decrypt(encrypted_text, shift):
return caesar_encrypt(encrypted_text, -shift)
decrypted = caesar_decrypt(encrypted, shift)
print(f"Decrypted text: {decrypted}")
```
阅读全文
相关推荐
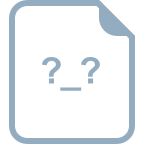
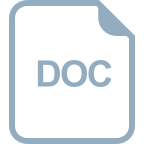
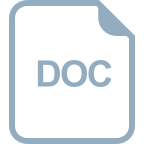
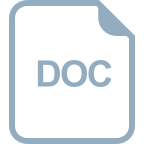










