org.springframework.data.redis.core.ValueOperations#setIfAbsent(K, V, java.time.Duration)
时间: 2023-12-11 18:03:34 浏览: 25
这是一个Redis操作,用于将给定的键值对存储到Redis数据库中。它的作用是仅当指定的key不存在时才设置它的值。如果key已经存在,则此操作不会执行任何操作,也不会更新现有值。
这个方法有三个参数:键,值和过期时间。如果过期时间不为null,则键值对将在指定的时间后过期并从Redis数据库中自动删除。如果过期时间为null,则键值对将永久保存在Redis数据库中。
需要注意的是,这个方法是线程安全的,因为Redis本身是单线程的,所以对Redis的操作都是线程安全的。
相关问题
org.springframework.data.redis.core.ValueOperations#increment 设置过期时间
是的,可以通过调用`ValueOperations#increment`方法的重载版本来设置过期时间。例如,使用以下代码可以将key的值递增1,并在60秒后过期:
```
ValueOperations<String, String> ops = redisTemplate.opsForValue();
ops.increment("key", 1L);
redisTemplate.expire("key", 60, TimeUnit.SECONDS);
```
其中,`redisTemplate`是RedisTemplate实例,类型参数分别为String和String。`ops.increment`方法的第二个参数指定递增的数量,这里为1L。`redisTemplate.expire`方法用于设置过期时间,第一个参数为key,第二个参数为过期时间,第三个参数为时间单位。
java: 程序包org.springframework.data.redis.core不存在\
您好!对于缺少程序包 "org.springframework.data.redis.core" 的问题,您可以尝试以下几个解决方案:
1. 确保您的项目中已经包含了 Redis 相关的依赖项。在 Maven 项目中,您可以在 pom.xml 文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
如果您使用的是 Gradle,可以在 build.gradle 文件中添加以下依赖项:
```groovy
implementation 'org.springframework.boot:spring-boot-starter-data-redis'
```
请注意,这只是一个示例依赖项,实际的版本可能会有所不同。建议根据您的项目需要进行适当的调整。
2. 如果您已经添加了正确的依赖项但仍然出现问题,请确保您的项目已经正确配置了 Spring Boot 并启用了 Redis 的自动配置。您可以在应用程序的主类上添加 `@EnableRedisRepositories` 注解,以启用 Redis 仓库功能。
3. 如果您正在使用的是非 Spring Boot 项目,或者上述解决方案无效,可能需要手动配置 Redis 相关的 Bean。您可以创建一个 Redis 连接工厂的 Bean,并将其注入到您的其他组件中。以下是一个示例配置类:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.jedis.JedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
JedisConnectionFactory jedisConnectionFactory = new JedisConnectionFactory();
// 设置 Redis 连接相关配置,如主机名、端口号、密码等
// jedisConnectionFactory.setHostName("localhost");
// jedisConnectionFactory.setPort(6379);
// jedisConnectionFactory.setPassword("password");
return jedisConnectionFactory;
}
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
return template;
}
}
```
请注意,上述示例中的 Redis 连接工厂配置是基于 Jedis 库的,您也可以根据您实际使用的 Redis 客户端库进行适当调整。
希望以上解决方案能够帮助到您!如果您有任何进一步的问题,请随时提问。
相关推荐
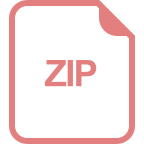
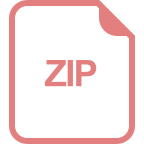
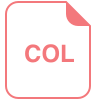
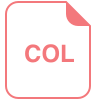
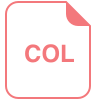
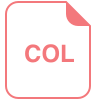








