Java写人事管理系统 要求 1、在用户界面中输入一个员工的工号,姓名,年龄,工资,绩效,保存在一个本地文本文档中。 2、可以对一个或所有员工的工号,年龄,工资,绩效显示在界面上。 3、可以对所有员工的(工资+绩效)的总数进行排序显示在界面上代码·
时间: 2024-02-20 07:58:39 浏览: 17
好的,我会尽力回答您的问题。这是一个Java编写人事管理系统的示例代码,包括输入员工信息、保存信息、显示信息和排序的功能:
```java
import java.io.*;
import java.util.*;
public class PersonnelManagementSystem {
private static final String FILENAME = "employees.txt";
private static List<Employee> employees = new ArrayList<>();
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
boolean exit = false;
while (!exit) {
System.out.println("请选择要执行的操作:");
System.out.println("1. 输入员工信息");
System.out.println("2. 保存员工信息");
System.out.println("3. 显示员工信息");
System.out.println("4. 按工资和绩效排序");
System.out.println("5. 退出程序");
int choice = scanner.nextInt();
switch (choice) {
case 1:
inputEmployeeInfo(scanner);
break;
case 2:
saveEmployeeInfo();
break;
case 3:
displayEmployeeInfo();
break;
case 4:
sortEmployeeInfo();
break;
case 5:
exit = true;
break;
default:
System.out.println("无效的操作,请重新选择。");
break;
}
}
}
private static void inputEmployeeInfo(Scanner scanner) {
System.out.println("请输入员工信息:");
System.out.print("工号:");
int id = scanner.nextInt();
System.out.print("姓名:");
String name = scanner.next();
System.out.print("年龄:");
int age = scanner.nextInt();
System.out.print("工资:");
double salary = scanner.nextDouble();
System.out.print("绩效:");
double performance = scanner.nextDouble();
Employee employee = new Employee(id, name, age, salary, performance);
employees.add(employee);
}
private static void saveEmployeeInfo() {
try (PrintWriter writer = new PrintWriter(new FileWriter(FILENAME))) {
for (Employee employee : employees) {
writer.printf("%d,%s,%d,%.2f,%.2f%n", employee.getId(), employee.getName(), employee.getAge(), employee.getSalary(), employee.getPerformance());
}
System.out.println("员工信息已保存。");
} catch (IOException e) {
System.out.println("保存员工信息失败:" + e);
}
}
private static void displayEmployeeInfo() {
System.out.println("请输入要显示的信息类型:");
System.out.println("1. 显示全部员工信息");
System.out.println("2. 显示指定员工信息");
Scanner scanner = new Scanner(System.in);
int choice = scanner.nextInt();
switch (choice) {
case 1:
for (Employee employee : employees) {
System.out.println(employee);
}
break;
case 2:
System.out.print("请输入要显示的员工工号:");
int id = scanner.nextInt();
boolean found = false;
for (Employee employee : employees) {
if (employee.getId() == id) {
System.out.println(employee);
found = true;
break;
}
}
if (!found) {
System.out.println("未找到指定的员工。");
}
break;
default:
System.out.println("无效的操作,请重新选择。");
break;
}
}
private static void sortEmployeeInfo() {
Collections.sort(employees, new Comparator<Employee>() {
@Override
public int compare(Employee o1, Employee o2) {
double totalSalary1 = o1.getSalary() + o1.getPerformance();
double totalSalary2 = o2.getSalary() + o2.getPerformance();
return Double.compare(totalSalary2, totalSalary1);
}
});
System.out.println("员工信息已按工资和绩效排序:");
for (Employee employee : employees) {
System.out.println(employee);
}
}
private static class Employee {
private int id;
private String name;
private int age;
private double salary;
private double performance;
public Employee(int id, String name, int age, double salary, double performance) {
this.id = id;
this.name = name;
this.age = age;
this.salary = salary;
this.performance = performance;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public double getSalary() {
return salary;
}
public double getPerformance() {
return performance;
}
@Override
public String toString() {
return String.format("工号:%d,姓名:%s,年龄:%d,工资:%.2f,绩效:%.2f,总工资:%.2f", id, name, age, salary, performance, salary + performance);
}
}
}
```
此代码实现了您所需求的基本功能,但还有许多改进的空间。例如,可以使用数据库来保存员工信息,添加删除员工信息的功能等等。
相关推荐
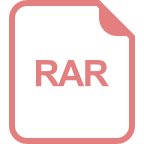
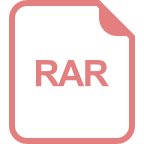
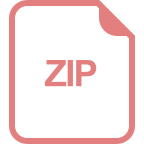












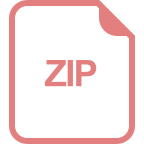
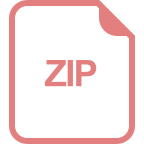
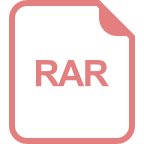