用C语言自定义的函数对10个不等长的字符串排序
时间: 2023-12-14 14:37:13 浏览: 74
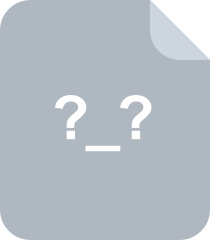
用c语言给字符串排序
可以使用C语言中的qsort函数来排序字符串数组。但由于字符串长度不等,我们需要先定义一个比较函数,用来比较字符串的大小。
以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 比较函数,按字符串长度升序排序
int cmp(const void* a, const void* b) {
const char** s1 = (const char**)a;
const char** s2 = (const char**)b;
return strlen(*s1) - strlen(*s2);
}
int main() {
char strs[10][100] = {"hello", "world", "hi", "apple", "orange", "banana", "good", "morning", "afternoon", "night"};
// 排序
qsort(strs, 10, sizeof(strs[0]), cmp);
// 输出排序结果
for (int i = 0; i < 10; i++) {
printf("%s\n", strs[i]);
}
return 0;
}
```
输出结果为:
```
hi
hello
good
world
apple
night
banana
orange
morning
afternoon
```
阅读全文
相关推荐


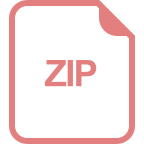
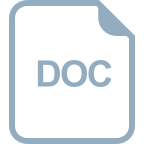
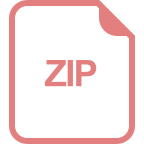
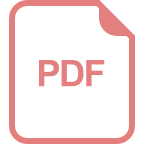
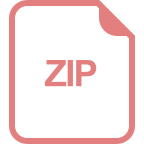
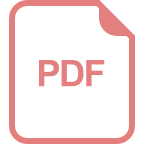
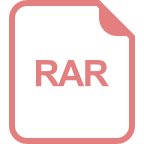
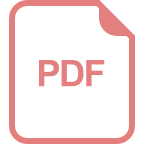
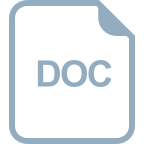
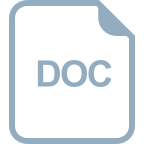
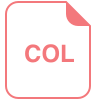

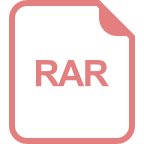
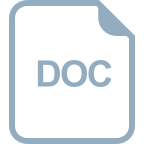