俄罗斯方块文件代码
时间: 2023-08-30 07:06:51 浏览: 75
以下是一个简单的C++俄罗斯方块游戏的文件代码示例,供您参考:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
const int ROWS = 20;
const int COLS = 10;
const int BLOCK_TYPES = 7;
const int BLOCK_SIZE = 4;
// 定义方块形状
const string BLOCK_SHAPES[BLOCK_TYPES][BLOCK_SIZE] = {
{
"....",
"####",
"....",
"...."
},
{
"....",
".##.",
".##.",
"...."
},
{
"....",
"##..",
".##.",
"...."
},
{
"....",
".##.",
"##..",
"...."
},
{
"....",
".#..",
"###.",
"...."
},
{
"....",
"###.",
".#..",
"...."
},
{
"....",
"..#.",
"###.",
"...."
}
};
// 定义方块颜色
const int BLOCK_COLORS[BLOCK_TYPES] = {
1, // 红
2, // 绿
3, // 蓝
4, // 黄
5, // 洋红
6, // 青
7 // 橙
};
// 定义游戏状态
enum GameState {
START,
PLAYING,
PAUSED,
GAMEOVER
};
// 定义游戏区域的坐标结构体
struct Coordinate {
int row;
int col;
};
// 定义游戏区域的方块结构体
struct Block {
Coordinate position;
string shape[BLOCK_SIZE];
int color;
};
// 定义游戏区域
Block gameArea[ROWS][COLS];
// 定义当前方块
Block currentBlock;
// 定义下一个方块
Block nextBlock;
// 定义游戏状态
GameState gameState = START;
// 定义分数
int score = 0;
// 生成随机方块
void generateRandomBlock(Block& block) {
int type = rand() % BLOCK_TYPES;
block.color = BLOCK_COLORS[type];
for (int i = 0; i < BLOCK_SIZE; i++) {
block.shape[i] = BLOCK_SHAPES[type][i];
}
block.position.row = 0;
block.position.col = COLS / 2 - 2;
}
// 判断是否可以移动方块
bool canMoveBlock(Block block, int rowOffset, int colOffset) {
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
if (block.shape[i][j] != '.') {
int row = block.position.row + i + rowOffset;
int col = block.position.col + j + colOffset;
if (row < 0 || row >= ROWS || col < 0 || col >= COLS || gameArea[row][col].color != 0) {
return false;
}
}
}
}
return true;
}
// 移动方块
void moveBlock(Block& block, int rowOffset, int colOffset) {
block.position.row += rowOffset;
block.position.col += colOffset;
}
// 旋转方块
void rotateBlock(Block& block) {
string temp[BLOCK_SIZE][BLOCK_SIZE];
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
temp[i][j] = block.shape[i][j];
}
}
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
block.shape[i][j] = temp[BLOCK_SIZE - j - 1][i];
}
}
}
// 固定方块
void fixBlock(Block block) {
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
if (block.shape[i][j] != '.') {
int row = block.position.row + i;
int col = block.position.col + j;
gameArea[row][col] = block;
}
}
}
}
// 消除行
void clearLines() {
int lines = 0;
for (int i = ROWS - 1; i >= 0; i--) {
bool full = true;
for (int j = 0; j < COLS; j++) {
if (gameArea[i][j].color == 0) {
full = false;
break;
}
}
if (full) {
lines++;
for (int k = i; k >= 1; k--) {
for (int j = 0; j < COLS; j++) {
gameArea[k][j] = gameArea[k - 1][j];
}
}
for (int j = 0; j < COLS; j++) {
gameArea[0][j].color = 0;
}
i++;
}
}
score += lines * 100;
}
// 判断游戏是否结束
bool isGameOver() {
for (int j = 0; j < COLS; j++) {
if (gameArea[0][j].color != 0) {
return true;
}
}
return false;
}
// 保存游戏状态到文件
void saveGame() {
ofstream file("game.dat");
if (file.is_open()) {
file << gameState << endl;
file << score << endl;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
file << gameArea[i][j].color << " ";
}
file << endl;
}
file.close();
}
}
// 从文件加载游戏状态
void loadGame() {
ifstream file("game.dat");
if (file.is_open()) {
int state;
file >> state;
gameState = (GameState)state;
file >> score;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int color;
file >> color;
gameArea[i][j].color = color;
}
}
file.close();
}
}
int main() {
srand(time(NULL));
// 生成下一个方块
generateRandomBlock(nextBlock);
while (true) {
if (gameState == START) {
cout << "Press [Enter] to start the game...";
cin.get();
gameState = PLAYING;
}
else if (gameState == PLAYING) {
if (canMoveBlock(currentBlock, 1, 0)) {
moveBlock(currentBlock, 1, 0);
}
else {
fixBlock(currentBlock);
clearLines();
currentBlock = nextBlock;
generateRandomBlock(nextBlock);
if (!canMoveBlock(currentBlock, 0, 0)) {
gameState = GAMEOVER;
}
}
system("cls");
// 输出游戏区域
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (gameArea[i][j].color == 0) {
cout << ".";
}
else {
cout << gameArea[i][j].color;
}
}
cout << endl;
}
cout << "Score: " << score << endl;
cout << "Next block:" << endl;
// 输出下一个方块
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
if (nextBlock.shape[i][j] == '.') {
cout << ".";
}
else {
cout << nextBlock.color;
}
}
cout << endl;
}
Sleep(100);
}
else if (gameState == GAMEOVER) {
cout << "Game over!" << endl;
cout << "Your score: " << score << endl;
saveGame();
break;
}
else if (gameState == PAUSED) {
cout << "Game paused. Press [Enter] to resume...";
cin.get();
gameState = PLAYING;
}
}
return 0;
}
```
这个示例代码中,主要包含了俄罗斯方块游戏的核心逻辑,包括方块的生成、移动、旋转、固定,游戏区域的维护,行的消除,游戏状态的判断,以及保存和加载游戏状态到文件等功能。这个示例代码较为简单,但是可以作为您实现一个更为完整的俄罗斯方块游戏的参考。
阅读全文
相关推荐
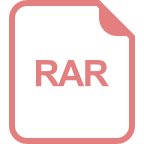
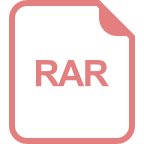
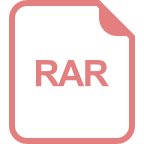
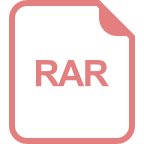
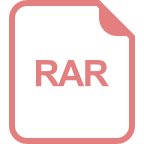
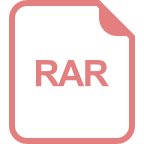
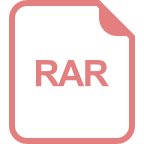
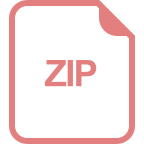
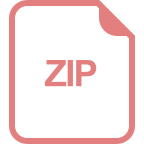
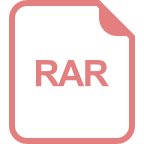
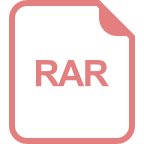
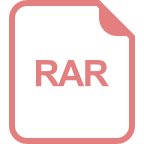
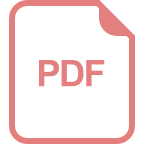