from netmiko import ConnectHandler import datetime fortigate = { 'device_type': 'fortinet', 'host': '10.254.254.254', 'username': 'backup',#username 'password': '********',#password } net_connect = ConnectHandler(**fortigate) output = net_connect.send_command('show full-configuration')output current_time = datetime.datetime.today().strftime('%Y_%b_%d')current_time with open('/Users/apple/Desktop/backup/' + str(fortigate['host']) + '_' + str(current_time) + '.cfg', 'w') as f: for line in output: f.write(line) 把这段代码用Linux C语言改写完善
时间: 2023-08-12 07:08:52 浏览: 165
这段Python代码的功能是连接到Fortigate设备,获取它的完整配置,并将其保存到文件中。以下是使用C语言实现相同功能的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <libssh/libssh.h>
#define BUFFER_SIZE 1024
int main() {
ssh_session session;
ssh_channel channel;
// Connect to Fortigate device
session = ssh_new();
if (session == NULL) {
printf("Error creating SSH session\n");
return 1;
}
ssh_options_set(session, SSH_OPTIONS_HOST, "10.254.254.254");
ssh_options_set(session, SSH_OPTIONS_USER, "backup");
ssh_options_set(session, SSH_OPTIONS_PASSWORD, "********");
if (ssh_connect(session) != SSH_OK) {
printf("Error connecting to Fortigate device: %s\n", ssh_get_error(session));
ssh_free(session);
return 1;
}
// Open shell channel
channel = ssh_channel_new(session);
if (channel == NULL) {
printf("Error creating SSH channel\n");
ssh_disconnect(session);
ssh_free(session);
return 1;
}
if (ssh_channel_open_session(channel) != SSH_OK) {
printf("Error opening SSH channel\n");
ssh_channel_free(channel);
ssh_disconnect(session);
ssh_free(session);
return 1;
}
// Send command to get full configuration
char command[BUFFER_SIZE] = "show full-configuration";
if (ssh_channel_request_exec(channel, command) != SSH_OK) {
printf("Error executing command: %s\n", ssh_get_error(session));
ssh_channel_close(channel);
ssh_channel_free(channel);
ssh_disconnect(session);
ssh_free(session);
return 1;
}
// Read output from command
char buffer[BUFFER_SIZE];
int nbytes;
FILE *fp;
char filename[BUFFER_SIZE];
time_t current_time = time(NULL);
struct tm *tm = localtime(¤t_time);
strftime(filename, BUFFER_SIZE, "/Users/apple/Desktop/backup/10.254.254.254_%Y_%b_%d.cfg", tm);
fp = fopen(filename, "w");
if (fp == NULL) {
printf("Error creating backup file\n");
ssh_channel_close(channel);
ssh_channel_free(channel);
ssh_disconnect(session);
ssh_free(session);
return 1;
}
while ((nbytes = ssh_channel_read(channel, buffer, BUFFER_SIZE, 0)) > 0) {
fwrite(buffer, nbytes, 1, fp);
}
fclose(fp);
// Clean up
ssh_channel_send_eof(channel);
ssh_channel_close(channel);
ssh_channel_free(channel);
ssh_disconnect(session);
ssh_free(session);
return 0;
}
```
这段代码使用libssh库连接到Fortigate设备并执行命令。它将命令的输出写入文件,文件名包括设备IP地址和备份日期。
阅读全文
相关推荐
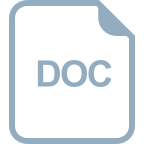





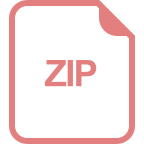
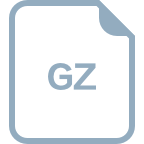
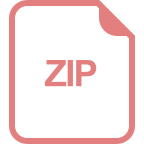
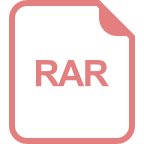








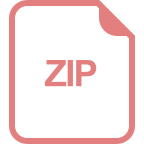