#include <iostream> #include <cstring> using namespace std; class Person { public: Person& setName(string nn) { name = nn; return *this; } Person& setAge(int a) { age = a; } void showPerson()const { cout << "This person is \"" << name << "\" whose age is " << age << "." << endl; } public: Person() { name = "no name";age = 0; cout << "A person whose name is \"" << name << "\" and age is " << age << " is created!" << endl; } Person(string nn) { name = nn; age = 0; cout << "A person whose name is \"" << name << "\" and age is " << age << " is created!" << endl; } Person(string s, int a) { name = s; age = a; cout << "A person whose name is \"" << name << "\" and age is " << age << " is created!" << endl; } Person(const Person & p) { name = p.name; age = p.age; cout << "A person whose name is \"" << name << "\" and age is " << age << " is cloned!" << endl; } ~Person() { cout << "A person whose name is \"" << name << "\" and age is " << age << " is erased!" << endl; } private: string name; int age; }; int main() { int cases; char str[80]; int age; Person noname, Tom("Tom", 16), anotherTom(Tom); cin>>cases; for (int ca = 0; ca < cases; ca++) { cin>>str>>age; Person newPerson(str, age); newPerson.showPerson(); } anotherTom.setName(str).setAge(18); anotherTom.showPerson(); noname.showPerson(); return 0; }
时间: 2024-03-08 16:45:32 浏览: 88
这是一段 C++ 代码,定义了一个名为 Person 的类,并在 main 函数中使用了该类。在 Person 类中,有四个构造函数用于创建对象,分别是默认构造函数、带一个参数的构造函数、带两个参数的构造函数和复制构造函数。同时,该类还定义了几个成员函数,包括 setName、setAge 和 showPerson,用于设置和展示 Person 对象的姓名和年龄信息。
在 main 函数中,首先创建了三个 Person 对象,分别是默认构造函数创建的 noname 对象、带一个参数的构造函数创建的 Tom 对象和复制构造函数创建的 anotherTom 对象。接着,根据输入的数据创建了若干个 Person 对象,并展示了它们的信息。最后,使用了链式调用的方式,对 anotherTom 对象的姓名和年龄进行了修改,并展示了其信息,以及 noname 对象的信息。
相关问题
4 IntelliSense: 没有与这些操作数匹配的 "<<" 运算符 操作数类型为: std::ostream << std::string f:\cpp_program\Polymorphic_call\Polymorphic_call\polymorach.cpp 13 8 Polymorphic_call 但是显示这个报错,如何解决
这个报错是因为在代码中使用了cout,但是没有包含iostream头文件。请确保在代码中包含`#include <iostream>`这一行,以便引入iostream库并使用cout。修改后的代码应该如下所示:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
Person(const char* name): _name(name)
{
}
// 虚函数
virtual void BuyTicket()
{
cout << _name << "Person:买票-全价 100¥" << endl;
}
protected:
string _name;
// int _id;
};
```
这样修改后,应该能够成功编译和运行代码。
阅读全文
相关推荐
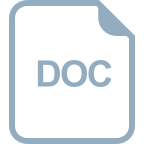
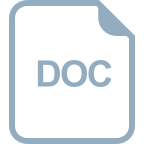
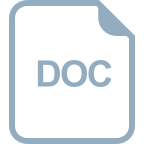
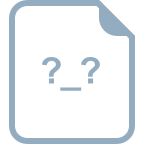
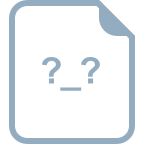
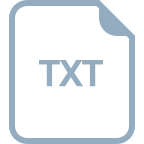
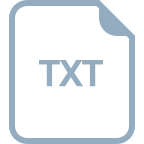
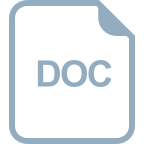
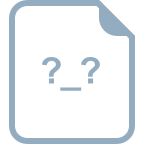
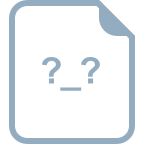
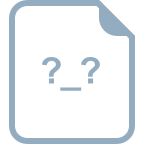
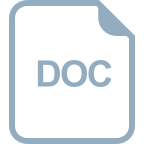
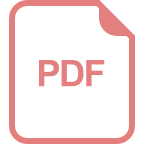
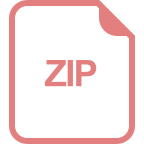
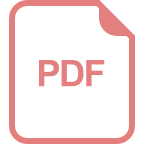
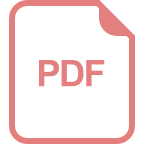
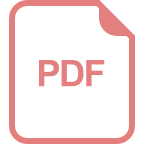
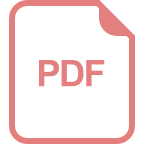