使用C语言完整写出使用while实现输入N输出1*2*3…N
时间: 2023-05-22 22:05:16 浏览: 492
非常欢迎你的提问!以下是使用C语言完整写出使用while实现输入N输出1*2*3…N的代码:
```
#include <stdio.h>
int main() {
int n, i = 1, result = 1;
printf("请输入N的值:");
scanf("%d", &n);
while (i <= n) {
result = result * i;
i++;
}
printf("1*2*3*...*N的值为:%d", result);
return 0;
}
```
如上代码所示,我们首先定义了变量n、i和result,分别作为输入的N值、循环的计数器和阶乘的结果。然后通过scanf函数获取用户输入的N的值。
接着,我们使用while循环,当i小于等于n时进行循环。在每一次循环中,我们更新result的值为之前的result与i的乘积,然后增加i的值。
当循环结束后,我们通过printf函数输出结果。
希望这个回答能够帮到你,如果有任何问题欢迎继续提问!
相关问题
c语言文件读写操作计算 A+B 输入 输入第一行是一个整数 N,代表接下来 N 行会有 N 组样例输入。 输出 每组输出占一行。使用while语句
在 C 语言中,如果你想通过文件读取两个整数 A 和 B,并计算它们的和,你可以按照以下步骤进行:
首先,你需要打开一个文件用于读取,如果文件不存在或无法打开,记得处理错误。然后,你可以使用 `fscanf` 函数从文件中逐行读取整数。
```c
#include <stdio.h>
int main() {
int n;
// 打开文件进行读取
FILE *file = fopen("input.txt", "r");
if (file == NULL) {
printf("Error opening file.\n");
return 1;
}
// 读取第一个整数 A
int a, b;
while (fscanf(file, "%d", &a) == 1) { // 检查是否成功读取
// 再次读取第二个整数 B
if (fscanf(file, "%d", &b) == 1) {
// 计算并输出 A + B 的结果
int sum = a + b;
printf("%d\n", sum);
} else {
printf("Failed to read second number.\n");
break; // 如果读取B失败,跳出循环
}
}
// 关闭文件
fclose(file);
return 0;
}
```
在这个例子中,假设数据存储在一个名为 "input.txt" 的文本文件中,每一行有两个整数。程序会依次读取每对数字,计算它们的和,并将结果打印到控制台。如果遇到文件读取错误或者格式不正确的数据,程序会给出相应的错误提示。
C语言 运用栈 写程序实现求解用户输入的表达式的值,例如输入1+2*3-16/2,则输出为-1
在C语言中,要实现一个简单的表达式求值器,我们可以利用栈数据结构来模拟计算过程。这里是一个基本的步骤:
1. 定义一个函数`evaluate_expression()`,接受用户输入的字符串作为参数。
2. 创建两个栈:一个用于存储操作数(整数值),另一个用于存储运算符及其优先级。
3. 遍历输入的表达式,遇到数字就压入操作数栈;遇到运算符则比较其优先级,并根据规则执行相应的操作。
4. 对于运算符,我们通常处理加法、减法、乘法和除法。遵循"左结合"的原则,即先处理最左边的相同优先级运算符。
5. 当遇到右括号时,意味着一个完整表达式的结束,从操作数栈弹出操作数,并计算结果直到遇到左括号。
6. 最终,当遍历完所有字符后,如果还有剩余的操作数,计算并返回最后一个结果。
下面是一个简化的伪代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
int precedence(char op) {
// 根据运算符的优先级定义,例如:'*' > '+' > '-' > '/'
}
void apply_operator(int *ops, int *ops_ptr, int *operators, int *operators_ptr) {
int b = *ops_ptr--;
int a = *ops_ptr--;
switch (*operators_ptr--) {
case '+':
*ops_ptr = a + b;
break;
case '-':
*ops_ptr = a - b;
break;
case '*':
*ops_ptr = a * b;
break;
case '/':
if (b != 0)
*ops_ptr = a / b;
else {
printf("Error: Division by zero");
return;
}
break;
}
}
int evaluate_expression(char *expression) {
stack_t ops; // 操作数栈
stack_t operators; // 运算符栈
for (int i = 0; expression[i]; ++i) {
if (isdigit(expression[i])) {
int num = 0;
while (isdigit(expression[i])) {
num = num * 10 + (expression[i] - '0');
++i;
}
push(ops, &num);
} else {
while (!is_empty(operators) && precedence(expression[i]) <= precedence(top(operators))) {
apply_operator(&top(ops), &ops_top, operators, &operators_top);
pop(operators);
}
push(operators, expression[i]);
}
}
while (!is_empty(operators)) {
apply_operator(&top(ops), &ops_top, operators, &operators_top);
pop(operators);
}
return top(ops); // 返回最终的结果
}
int main() {
char expression[] = "1+2*3-16/2";
int result = evaluate_expression(expression);
printf("Result: %d\n", result);
return 0;
}
```
请注意,这个例子是一个简化版,实际实现中需要考虑错误处理(如缺少闭合括号等)以及更复杂的情况,比如支持负数、小数和科学计数法表示的数字。此外,这个代码片段仅做教学演示,可能需要优化和调整以适应实际项目需求。
阅读全文
相关推荐
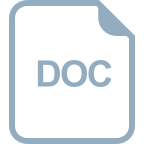
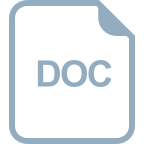
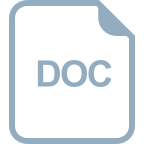














