C51中lcd一直在接收串口的数字,怎样将一直接收的不同长度的数字显示在lcd上,每次接收的数字要在上一次接收的后面
时间: 2023-12-03 20:43:05 浏览: 25
首先,你需要使用串口中断来接收数字,这样可以避免一直占用CPU资源。每当接收到数字时,将其存储到一个缓冲区中,并记录数字的长度。然后,你可以使用LCD显示库来显示这些数字。
以下是示例代码:
```c
#include <reg51.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LCD_DB P0
sbit RS = P2^0;
sbit EN = P2^1;
#define BUFFER_SIZE 20
volatile unsigned char buffer[BUFFER_SIZE]; // 接收缓冲区
volatile unsigned char buffer_len = 0; // 缓冲区中数字的长度
void lcd_init();
void lcd_clear();
void lcd_write_string(unsigned char row, unsigned char col, char* str);
void lcd_write_char(unsigned char row, unsigned char col, char c);
void lcd_write_cmd(unsigned char cmd);
void lcd_write_data(unsigned char data);
void uart_init();
void uart_isr() interrupt 4;
void main() {
lcd_init(); // 初始化LCD
uart_init(); // 初始化串口
EA = 1; // 开启总中断
while (1) {} // 保持程序运行
}
void uart_init() {
TMOD = 0x20; // 配置为定时器1工作在方式2,自动重装载
TH1 = 0xFD; // 波特率为9600,定时器1初值
TL1 = TH1; // 定时器1初值
TR1 = 1; // 启动定时器1
SCON = 0x50; // 串口工作在模式1,允许接收
ES = 1; // 开启串口中断
}
void uart_isr() interrupt 4 {
if (RI) { // 接收到数据
RI = 0; // 清除接收标志位
unsigned char data = SBUF; // 读取串口数据
if (buffer_len < BUFFER_SIZE - 1) { // 缓冲区未满
buffer[buffer_len++] = data; // 存储数据
buffer[buffer_len] = '\0'; // 添加字符串结束符
}
}
}
void lcd_init() {
lcd_write_cmd(0x38); // 设置16*2显示,5*7点阵,8位数据总线
lcd_write_cmd(0x0C); // 打开显示,关闭光标
lcd_write_cmd(0x06); // 光标右移,字符不移动
lcd_write_cmd(0x01); // 清屏
}
void lcd_clear() {
lcd_write_cmd(0x01); // 清屏
}
void lcd_write_string(unsigned char row, unsigned char col, char* str) {
unsigned char pos = row * 0x40 + col; // 计算显示位置
lcd_write_cmd(pos | 0x80); // 设置DDRAM地址
while (*str) { // 写入字符串
lcd_write_data(*str++);
}
}
void lcd_write_char(unsigned char row, unsigned char col, char c) {
unsigned char pos = row * 0x40 + col; // 计算显示位置
lcd_write_cmd(pos | 0x80); // 设置DDRAM地址
lcd_write_data(c); // 写入字符
}
void lcd_write_cmd(unsigned char cmd) {
RS = 0; // 命令模式
LCD_DB = cmd; // 写入命令
EN = 1;
EN = 0;
}
void lcd_write_data(unsigned char data) {
RS = 1; // 数据模式
LCD_DB = data; // 写入数据
EN = 1;
EN = 0;
}
```
在主程序中,我们初始化了LCD和串口,并开启了总中断。在串口中断中,我们读取接收到的数字,并将其存储到缓冲区中。在LCD显示函数中,我们根据行和列计算出显示位置,并将字符串或字符写入该位置。
你可以在主程序中使用以下代码来显示缓冲区中的数字:
```c
lcd_write_string(0, 0, "Received: ");
lcd_write_string(1, 0, buffer);
```
这将在LCD的第一行显示"Received: ",并在第二行显示缓冲区中的数字。每当接收到新的数字时,LCD将更新显示。
相关推荐
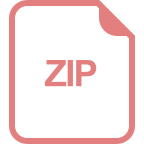














