mpu6050的DMP
时间: 2023-07-25 21:04:01 浏览: 64
首先,需要在STM32CubeMX中添加MPU6050的驱动程序,并配置I2C接口。然后,需要将MPU6050的DMP库文件添加到工程中,并在代码中调用相关函数来初始化和使用DMP功能。具体的移植步骤可以参考MPU6050的官方文档和STM32CubeMX的使用手册。需要注意的是,移植过程中可能会遇到一些问题,需要根据具体情况进行调试和解决。
相关问题
mpu6050 dmp下载
MPU6050是一款集成了3轴加速度计和3轴陀螺仪传感器的模块,具有测量加速度和角速度的功能。DMP(数字运动处理器)是MPU6050内部集成的处理器,可以用于实时处理传感器数据,并提供准确的姿态估计。
要进行MPU6050 DMP的下载,首先需要进行硬件接线。将MPU6050连接到Arduino开发板上,确保电源和数据线连接正确。
接下来,需要下载I2Cdev库和MPU6050库。这两个库都是开源的,可以从GitHub或开发者社区下载。将这两个库放入Arduino IDE的库文件夹中,然后打开Arduino IDE。
在Arduino IDE中,首先打开MPU6050_DMP6例程。这个例程包含了用于驱动MPU6050 DMP的相关代码。点击“文件”->“示例”->“MPU6050_DMP6”打开例程。
在打开的例程中,选择对应的Arduino开发板和端口,然后编译并上传代码到Arduino开发板。上传完成后,打开串口监视器。
在串口监视器中,将波特率设置为115200,并点击“重置软件模拟器”。软件模拟器会开始模拟MPU6050的输出数据,并通过串口打印出来。
通过串口监视器,我们可以看到MPU6050的加速度和角速度数据,以及经过DMP处理后的姿态估计(如俯仰角、横滚角和航向角)。
至此,MPU6050 DMP的下载已完成。你可以根据自己的需求,修改和应用这个例程,以实现更复杂的功能,如姿态控制、运动跟踪等。
MPU6050 DMP使用
MPU6050 DMP是指使用MPU6050的数字运动处理器(DMP)功能。通过结合MPU6050的DMP,可以将原始数据直接转换成四元数输出,从而方便地计算出欧拉角(yaw、roll和pitch)。\[1\]要使用MPU6050 DMP,首先需要新建一个STM32CubeMX工程,并插入MPU6050和DMP的初始化代码。在初始化MPU6050之后,可以通过DMP获取四元数数据,然后计算出欧拉角。\[2\]在代码中需要定义使用的传感器为MPU6050,并指定驱动部分为MSP430的驱动(移植到STM32F1)。\[3\]
#### 引用[.reference_title]
- *1* *2* [STM32F103RCT6——MPU6050使用](https://blog.csdn.net/northern_light_/article/details/130835142)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [STM32 使用DMP库处理MPU6050数据](https://blog.csdn.net/qq_44011116/article/details/113738183)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
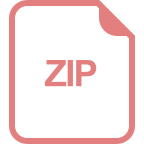













