创建无向图的邻接表c语言
时间: 2023-12-03 19:03:46 浏览: 42
以下是创建无向图的邻接表的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int vertex;
struct Node* next;
};
struct Graph {
int numVertices;
struct Node** adjLists;
};
struct Node* createNode(int v) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
struct Graph* createGraph(int vertices) {
struct Graph* graph = (struct Graph*)malloc(sizeof(struct Graph));
graph->numVertices = vertices;
graph->adjLists = (struct Node**)malloc(vertices * sizeof(struct Node*));
int i;
for (i = 0; i < vertices; i++)
graph->adjLists[i] = NULL;
return graph;
}
void addEdge(struct Graph* graph, int src, int dest) {
// Add edge from src to dest
struct Node* newNode = createNode(dest);
newNode->next = graph->adjLists[src];
graph->adjLists[src] = newNode;
// Add edge from dest to src
newNode = createNode(src);
newNode->next = graph->adjLists[dest];
graph->adjLists[dest] = newNode;
}
void printGraph(struct Graph* graph) {
int v;
for (v = 0; v < graph->numVertices; v++) {
struct Node* temp = graph->adjLists[v];
printf("\n Adjacency list of vertex %d\n head ", v);
while (temp) {
printf("-> %d", temp->vertex);
temp = temp->next;
}
printf("\n");
}
}
int main() {
struct Graph* graph = createGraph(4);
addEdge(graph, 0, 1);
addEdge(graph, 0, 2);
addEdge(graph, 1, 2);
addEdge(graph, 2, 3);
printGraph(graph);
return 0;
}
```
这个例子中,我们创建了一个无向图的邻接表,它包含4个顶点。我们使用`createGraph`函数创建一个名为`graph`的指向图的结构体的指针。然后,我们使用`addEdge`函数添加顶点之间的边。最后,我们使用`printGraph`函数打印邻接表。
这个例子中的无向图如下所示:
```
1
/ \
0 2
\ /
3
```
打印出来的邻接表如下所示:
```
Adjacency list of vertex 0
head -> 2-> 1
Adjacency list of vertex 1
head -> 2-> 0
Adjacency list of vertex 2
head -> 3-> 1-> 0
Adjacency list of vertex 3
head -> 2
```
相关推荐













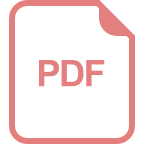