将两个递增的有序链表合并为一个递增的有序链表。要求结果链表仍使用原来两个链表的存储空间,不另外占用其他的存储空间。表中不允许有重复的数据。的C语言代码
时间: 2024-06-15 14:08:10 浏览: 65
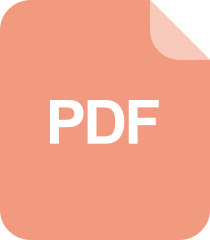
有两张单调递增有序的线性表A和B-采用顺序存储结构-将这两张表合并成C表-要求C表单调递减有序。Wo.pdf
可以使用双指针法来合并两个递增的有序链表。具体的C语言代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode* next;
};
// 合并两个递增的有序链表
struct ListNode* mergeTwoLists(struct ListNode* l1, struct ListNode* l2) {
// 创建一个虚拟头节点,方便操作
struct ListNode* dummy = (struct ListNode*)malloc(sizeof(struct ListNode));
dummy->next = NULL;
struct ListNode* cur = dummy;
// 双指针法合并链表
while (l1 && l2) {
if (l1->val <= l2->val) {
cur->next = l1;
l1 = l1->next;
} else {
cur->next = l2;
l2 = l2->next;
}
cur = cur->next;
}
// 将剩余的节点连接到结果链表中
cur->next = l1 ? l1 : l2;
// 释放虚拟头节点
struct ListNode* result = dummy->next;
free(dummy);
return result;
}
// 创建链表节点
struct ListNode* createNode(int val) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = val;
node->next = NULL;
return node;
}
// 打印链表
void printList(struct ListNode* head) {
struct ListNode* cur = head;
while (cur) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
}
int main() {
// 创建两个有序链表
struct ListNode* l1 = createNode(1);
l1->next = createNode(3);
l1->next->next = createNode(5);
struct ListNode* l2 = createNode(2);
l2->next = createNode(4);
l2->next->next = createNode(6);
// 合并两个有序链表
struct ListNode* mergedList = mergeTwoLists(l1, l2);
// 打印合并后的链表
printList(mergedList);
// 释放链表内存
struct ListNode* cur = mergedList;
while (cur) {
struct ListNode* temp = cur;
cur = cur->next;
free(temp);
}
return 0;
}
```
阅读全文
相关推荐
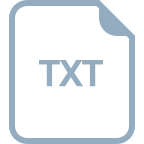
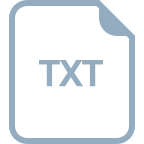
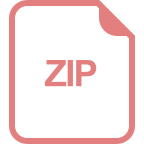
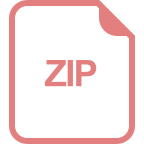
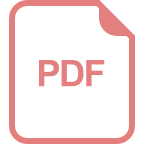
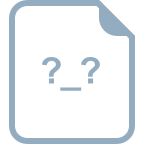
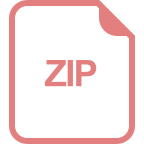
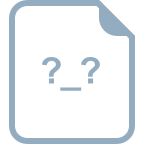
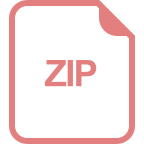
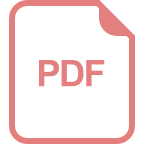