java如何下载// 在这里处理GeoJSON数据和其他参数 System.out.println("GeoJSON: " + geoJson); System.out.println("Param1: " + param1); System.out.println("Param2: " + param2); return new ResponseEntity<>("GeoJSON and params received", HttpStatus.OK);
时间: 2024-02-26 11:55:47 浏览: 25
Java下载可以使用Java自带的URL和URLConnection类,代码示例:
```java
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.URL;
import java.net.URLConnection;
public class DownloadExample {
public static void main(String[] args) {
try {
URL url = new URL("http://example.com/file.txt");
URLConnection conn = url.openConnection();
InputStream in = conn.getInputStream();
FileOutputStream out = new FileOutputStream("file.txt");
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) != -1) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("File downloaded");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
其中,URL类表示一个统一资源定位符,URLConnection类表示一个URL连接,可以使用它获取输入流和输出流。在示例中,我们通过输入流读取文件内容,通过输出流写入到本地文件中。
对于处理GeoJSON和其他参数,可以使用Java的JSON解析库,比如Google的Gson库。代码示例:
```java
import com.google.gson.Gson;
import com.google.gson.JsonObject;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
public class GeoJsonExample {
public ResponseEntity<String> processGeoJson(String geoJson, String param1, String param2) {
Gson gson = new Gson();
JsonObject geoJsonObj = gson.fromJson(geoJson, JsonObject.class);
System.out.println("GeoJSON: " + geoJsonObj.toString());
System.out.println("Param1: " + param1);
System.out.println("Param2: " + param2);
return new ResponseEntity<>("GeoJSON and params received", HttpStatus.OK);
}
}
```
其中,使用Gson库将GeoJSON字符串转为JsonObject对象,然后打印出来。
相关推荐
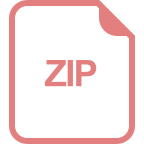
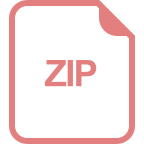














