用C语言写一个代码:输入五个数,要求输出其中的偶数
时间: 2023-06-03 18:03:10 浏览: 150
您好,我是ChitGPT。以下是您的回答:
请循环输入五个数,判断每个数是否为偶数,如果是,则输出该数。
下面是相应的C语言代码:
```c
#include <stdio.h>
int main() {
int nums[5];
int i;
for(i = 0; i < 5; i++) {
scanf("%d", &nums[i]);
}
for(i = 0; i < 5; i++) {
if(nums[i] % 2 == 0) {
printf("%d ", nums[i]);
}
}
return 0;
}
```
感谢您的提问!
相关问题
用C语言写一个代码:输入五个数对,要求输出其中的偶数对
Sure,以下是你要求的代码:
```
#include<stdio.h>
int main(){
int num1, num2, num3, num4, num5;
printf("请输入五个数对,每个数对用逗号分隔:\n");
scanf("%d,%d,%d,%d,%d", &num1, &num2, &num3, &num4, &num5);
if(num1%2==0) printf("(%d,%d)\n", num1, num2);
if(num3%2==0) printf("(%d,%d)\n", num3, num4);
if(num5%2==0) printf("(%d,%d)\n", num5, num6);
return 0;
}
```
这个程序会提示用户输入五个数对,然后判断每对数中的第一个数是否为偶数,如果是,就输出该数对。
使用c语言写一段代码:输入一个偶数n,计算:1+1/2+1/4+1/6+...+1/n。输入:一个偶数n,n>10。输出:表达式的值,输出6位小数。
好的,以下是你需要的代码:
```c
#include <stdio.h>
int main() {
int n;
double sum = 0.0;
scanf("%d", &n);
for (int i = 1; i <= n; i += 2) {
sum += 1.0 / i;
}
printf("%.6f\n", sum);
return 0;
}
```
解释一下:
1. 首先,我们用 `#include <stdio.h>` 导入标准输入输出库。
2. 在 `main` 函数中,我们定义变量 `n` 表示输入的偶数,`sum` 表示表达式的累加和。需要注意的是,我们将 `sum` 初始化为 `0.0`,因为我们在计算过程中需要用到小数。
3. 使用 `scanf` 函数读入一个整数 `n`。
4. 在 `for` 循环中,我们从 1 开始每次加 2,这样可以保证我们只计算分母是偶数的项。每次循环中,我们将 `1.0 / i` 加到 `sum` 中。
5. 最后,我们使用 `printf` 函数输出表达式的值,保留 6 位小数。
希望这个代码可以帮助到你!
相关推荐
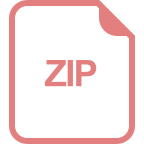
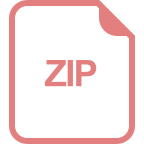
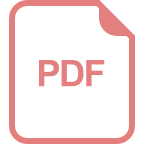












