请用Python帮我编写一个程序可以访问mqtt服务器,并将获取的数据存储到mysql数据库
时间: 2023-05-18 14:05:26 浏览: 297
以下是一个Python程序,可以连接到MQTT服务器并将获取的数据存储到MySQL数据库中:
```python
import paho.mqtt.client as mqtt
import mysql.connector
# MQTT服务器信息
mqtt_broker = "mqtt.example.com"
mqtt_port = 1883
mqtt_topic = "mytopic"
# MySQL数据库信息
mysql_host = "localhost"
mysql_user = "root"
mysql_password = "password"
mysql_database = "mydatabase"
# 连接到MySQL数据库
mysql_conn = mysql.connector.connect(
host=mysql_host,
user=mysql_user,
password=mysql_password,
database=mysql_database
)
mysql_cursor = mysql_conn.cursor()
# 定义MQTT消息处理函数
def on_message(client, userdata, message):
# 将消息转换为字符串
payload = message.payload.decode("utf-8")
# 将消息插入到MySQL数据库中
mysql_cursor.execute("INSERT INTO mytable (message) VALUES (%s)", (payload,))
mysql_conn.commit()
# 连接到MQTT服务器
mqtt_client = mqtt.Client()
mqtt_client.connect(mqtt_broker, mqtt_port)
mqtt_client.subscribe(mqtt_topic)
mqtt_client.on_message = on_message
# 开始循环处理MQTT消息
mqtt_client.loop_forever()
```
在上面的代码中,我们使用了paho-mqtt库来连接到MQTT服务器,并使用mysql-connector库来连接到MySQL数据库。我们定义了一个MQTT消息处理函数`on_message`,当接收到MQTT消息时,该函数将消息转换为字符串,并将其插入到MySQL数据库中。最后,我们连接到MQTT服务器并开始循环处理MQTT消息。
阅读全文
相关推荐
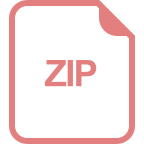

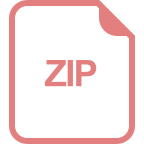
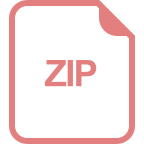
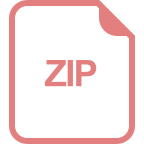


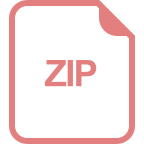
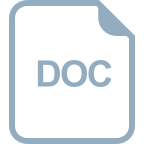
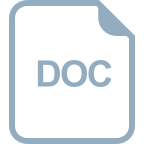
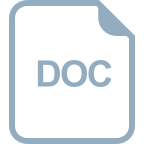
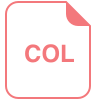
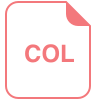
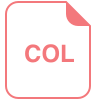
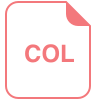
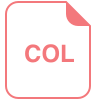
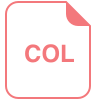