def spiral_search(center, radius, step): x, y = center yield x, y for r in range(1, radius + step, step): for i, (dx, dy) in enumerate(((1, 0), (0, 1), (-1, 0), (0, -1))): for j in range(r - (i % 2)): x += dx y += dy yield x, y # 定义建筑物遮蔽区域提取函数 def extract_building_shadow(image, dsm, ground_spacing, radius): shadow_mask = np.zeros_like(image, dtype=np.bool) for i in range(0, image.shape[0], ground_spacing): for j in range(0, image.shape[1], ground_spacing): if not np.any(shadow_mask[i, j]): center = (i, j) ground_height = dsm[i, j] for x, y in spiral_search(center, radius, ground_spacing): if x < 0 or x >= image.shape[0] or y < 0 or y >= image.shape[1]: continue if np.any(shadow_mask[x, y:]): continue height = dsm[x, y] if height > ground_height: shadow_mask[x, y] = True elif height == ground_height: if np.linalg.norm(np.array([x, y]) - np.array(center)) < \ np.linalg.norm(np.array([i, j]) - np.array(center)): shadow_mask[x, y] = True return shadow_mask这段代码是什么意思
时间: 2024-02-10 13:22:27 浏览: 108
这段代码是一个建筑物遮蔽区域提取的函数,其功能是根据遮挡高度判断建筑物遮蔽区域。具体来说,该函数输入为一幅遥感图像和一个数字表面模型(DSM),以及一个地面分辨率和一个搜索半径。函数的输出是一个布尔类型的遮蔽掩膜,其中为 True 的像素表示该处为建筑物遮蔽区域,为 False 的像素表示该处不是建筑物遮蔽区域。该函数的实现过程是:首先,以给定的地面分辨率为步长,对图像进行网格化,然后在每个网格的中心点上进行遮蔽区域判断。接着,以每个中心点为起点,按照螺旋线的方式向外搜索,并计算每个搜索点的高度。如果某个搜索点的高度大于该点所在中心点的高度,则将该搜索点标记为遮蔽区域。如果某个搜索点的高度等于该点所在中心点的高度,则比较该点到中心点的距离和该中心点到原始中心点的距离的大小,如果前者小于后者,则将该搜索点标记为遮蔽区域。最后,返回遮蔽掩膜。
相关问题
def spiral_search(center, radius, step): x, y = center yield x, y for r in range(1, radius + step, step): for i, (dx, dy) in enumerate(((1, 0), (0, 1), (-1, 0), (0, -1))): for j in range(r - (i % 2)): x += dx y += dy yield x, y这段代码是什么意思
这段代码是一个生成器函数,用于在给定中心点周围生成一系列坐标点。它实现了一种螺旋搜索算法,该算法将半径内的每个像素点遍历一遍。该算法从中心点开始,并沿着水平、竖直、水平、竖直的方向顺序移动。然后,它沿着螺旋路径向外扩散,每次移动一个步长。在每个步骤中,生成器函数先返回当前坐标点(即yield x, y),然后计算下一个坐标点并继续迭代,直到达到指定的半径。最终,该函数将返回一系列坐标点,这些点组成了从给定中心点开始的螺旋路径。
def extract_building_shadow(image, dsm, ground_spacing, radius): shadow_mask = np.zeros_like(image, dtype=np.bool) for i in range(0, image.shape[0], ground_spacing): for j in range(0, image.shape[1], ground_spacing): if not np.any(shadow_mask[i, j]): center = (i, j) ground_height = dsm[i, j] for x, y in spiral_search(center, radius, ground_spacing): if x < 0 or x >= image.shape[0] or y < 0 or y >= image.shape[1]: continue if np.any(shadow_mask[x, y:]): continue height = dsm[x, y] if height > ground_height: shadow_mask[x, y] = True elif height == ground_height: if np.linalg.norm(np.array([x, y]) - np.array(center)) < \ np.linalg.norm(np.array([i, j]) - np.array(center)): shadow_mask[x, y] = True return shadow_mask image = cv2.imread('C:\yingxiang\DJI_20230322140516_0026_V.JPG') dsm_path = 'C:/sanwei/jianmo/Productions/Production_2/Production_2_DSM_part_2_2.tif' dsm_dataset = gdal.Open(dsm_path) output_path = 'C:\yingxiang\shadow_mask.png'这段代码怎么改可以将掩膜结果保存
您可以在函数返回结果时添加以下代码将掩膜结果保存为 PNG 文件:
```
cv2.imwrite(output_path, shadow_mask.astype(np.uint8) * 255)
```
这将把 `shadow_mask` 数组中的布尔值转换为 0 和 1 的整数,并将其乘以 255 然后转换为无符号 8 位整数,最后使用 OpenCV 的 `imwrite()` 函数将其保存为 PNG 文件。请确保 `output_path` 变量包含正确的文件路径和文件名。
阅读全文
相关推荐
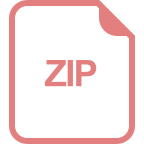
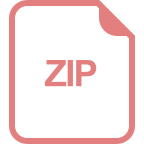
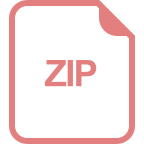



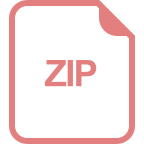
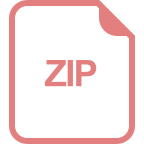