public class Program { static void Main(string[] args) { var customer1 = new Customer(1, "张三", "男"); Stack<Customer> customerstack = new Stack<Customer>(); //入栈 for (int index = 0; index < 6; index++) customerstack.Push(new Customer(index,"王"+index,"man")); //遍历所有栈内元素 foreach (Customer customer in customerstack) { Console.WriteLine("id is {0},name is {1}", customer.ID, customer.Name); } //出栈 Customer outCustomer = customerstack.Pop(); Console.WriteLine("正在出栈的是:" + outCustomer.Name); Console.WriteLine("当前栈内元素数量为:" + customerstack.Count); Console.ReadKey(); } }怎么实现按下一个案件继续执行打印操作
时间: 2024-02-14 15:24:46 浏览: 63
要实现按下一个按键后继续执行打印操作,你可以在代码中添加一个方法来处理按键事件,并在该方法中执行打印操作。以下是示例代码:
```csharp
public class Program
{
static void Main(string[] args)
{
var customer1 = new Customer(1, "张三", "男");
Stack<Customer> customerstack = new Stack<Customer>();
//入栈
for (int index = 0; index < 6; index++)
customerstack.Push(new Customer(index, "王" + index, "man"));
//遍历所有栈内元素
foreach (Customer customer in customerstack)
{
Console.WriteLine("id is {0}, name is {1}", customer.ID, customer.Name);
}
//按下一个按键后继续执行打印操作
Console.WriteLine("按下任意键继续执行打印操作...");
Console.ReadKey();
//出栈
Customer outCustomer = customerstack.Pop();
Console.WriteLine("正在出栈的是:" + outCustomer.Name);
Console.WriteLine("当前栈内元素数量为:" + customerstack.Count);
Console.ReadKey();
}
}
```
在上述代码中,我们在遍历栈内元素之后添加了一段代码,提示用户按下任意键继续执行打印操作。然后使用 `Console.ReadKey()` 来等待用户按下键盘上的任意键。当用户按下键后,程序会继续执行出栈操作和打印栈内元素数量的操作。
阅读全文
相关推荐
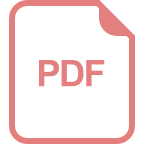
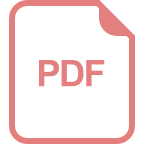
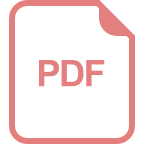
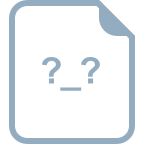
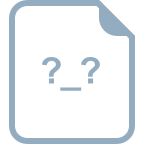
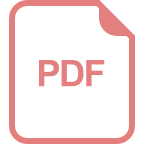
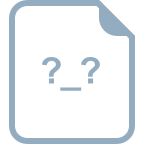
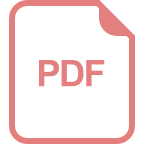
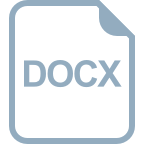
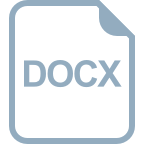
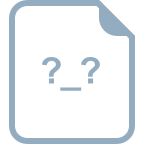
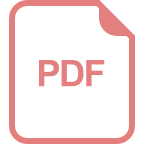
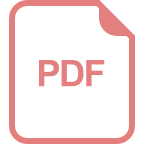
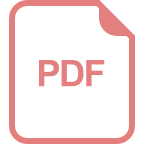
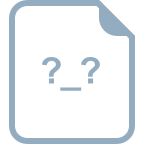
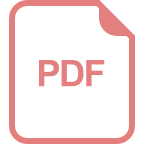
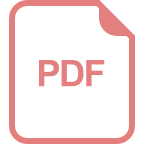
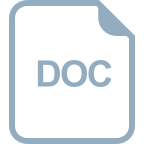