请输入一行字符,请统计所有字符中字母的个数,数字字符的个数,以及其它字符的个数C语言
时间: 2023-06-08 17:06:54 浏览: 81
实现如下:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char line[100];
int letters = 0, digits = 0, others = 0;
printf("请输入一行字符:");
fgets(line, 100, stdin);
for (int i = 0; line[i] != '\0'; i++) {
if (isalpha(line[i])) {
letters++;
} else if (isdigit(line[i])) {
digits++;
} else {
others++;
}
}
printf("字母个数:%d,数字个数:%d,其它字符个数:%d\n", letters, digits, others);
return 0;
}
```
该程序通过 `fgets` 函数获取输入的一行数据,并通过 `isalpha` 和 `isdigit` 函数判断每个字符的类型,最后统计出字母个数、数字个数和其它字符个数。
相关问题
用C语言编程实现,输入一行字符,分别统计出其中英文字母,空格,数字和其它字符的个数。编程实现,输入一行字符,分别统计出其中英文字母,空格,数字和其它字符的个数。
好的,这是一个很常见的编程问题,以下是一个简单的 C 语言代码实现:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i, letters, spaces, digits, others;
letters = spaces = digits = others = 0;
printf("请输入一行字符:\n");
gets(str);
for (i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i])) {
letters++;
} else if (isspace(str[i])) {
spaces++;
} else if (isdigit(str[i])) {
digits++;
} else {
others++;
}
}
printf("输入的字符串中包含:\n");
printf("%d 个字母\n", letters);
printf("%d 个空格\n", spaces);
printf("%d 个数字\n", digits);
printf("%d 个其他字符\n", others);
return 0;
}
```
这个程序会提示用户输入一行字符,然后使用 for 循环遍历字符串中的每个字符,使用 isalpha、isspace 和 isdigit 函数判断字符的类型,最后统计出每种类型的字符的个数并输出。
用C语言 输入一行字符,分别统计出其中英文字母、数字、空格和其他字符的个数。 输入 一行字符 输出 统计值
以下是两种C语言统计字符的例子:
1.常规计算
```c
#include <stdio.h>
int main(){
char c;
int letters=0,space=0,digit=0,other=0;
printf("请输入一行字符:\n");
while((c=getchar())!='\n'){
if(c>='a'&&c<='z'||c>='A'&&c<='Z') letters++;
else if(c==' ') space++;
else if(c>='0'&&c<='9') digit++;
else other++;
}
printf("字母数:%d\n空格数:%d\n数字数:%d\n其他字符数:%d",letters,space,digit,other);
return 0;
}
```
2.普通函数调用
```c
#include <stdio.h>
#include <string.h>
void count(char c[]){
int letters=0,space=0,digit=0,other=0;
int i;
for (i = 0; c[i]; i++) {
if(c[i]>='a'&&c[i]<='z'||c[i]>='A'&&c[i]<='Z') letters++;
else if(c[i]==' ') space++;
else if(c[i]>='0'&&c[i]<='9') digit++;
else other++;
}
printf("字母数:%d\n空格数:%d\n数字数:%d\n其他字符数:%d",letters,space,digit,other);
}
int main(){
char c[32];
printf("请输入一行字符:\n");
gets(c);
count(c);
return 0;
}
```
阅读全文
相关推荐
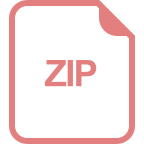













