vue中获取日期传到后端接口时,日期会少8个小时
时间: 2024-12-21 20:28:59 浏览: 11
在Vue中,当你将JavaScript Date对象发送到后端服务器,特别是在跨时区的情况下,可能会遇到时间差异的问题。默认情况下,Node.js(后端常用的语言环境)处理的时间是基于UTC(协调世界时),而浏览器中的日期通常是按照用户所在的本地时区计算的。
当直接从浏览器序列化并发送Date对象时,如果没有进行适当的转换,服务器接收到的日期就会比客户端显示的少8小时(如果是夏令时调整后的时差)。这是因为浏览器会考虑夏令时期间的额外一小时,而服务器不会自动加上这个小时。
为了防止这种问题,你可以采取以下步骤:
1. **客户端转换**:在发送数据前,使用`Date.prototype.toISOString()`或`moment.js`等库将日期标准化为ISO格式,并包含时区信息(如Zulu时间`Z`或`+00:00`)。
```javascript
const formattedDate = new Date().toISOString();
```
2. **后端解析**:在后端,你需要解析这个字符串,确保正确处理时区信息。大多数现代后端框架(如Express、Django、Spring Boot等)都有相应的中间件或函数可以处理这样的时间戳。
```javascript
// Node.js 示例
const date = new Date(req.body.date);
date.setUTCHours(date.getUTCHours() + 8); // 如果需要加8小时
```
相关问题
vue中获取日期传到后端接口时,日期会少8个小时moment.js如何解决
在Vue中,如果你使用了`moment.js`处理日期并在发送到后端接口时遇到日期减少8个小时的问题,这通常是因为服务器和前端的时间zone设置不同导致的。服务器可能使用的是UTC或其他非客户端时间,而默认的`moment.js`创建的日期是基于客户端时间的。
为了解决这个问题,你可以按照以下步骤操作:
1. **转换时区**:
使用`moment-timezone`库,它允许你轻松地处理时区。首先,你需要安装这个依赖:
```
npm install moment-timezone
```
然后,在发送请求前,将日期转换成后端期望的时区:
```javascript
import moment from 'moment-timezone';
const clientDate = moment(date, "YYYY-MM-DD HH:mm:ss"); // 假设date是你本地存储的日期
const serverTimezone = 'Asia/Shanghai'; // 后端使用的时区
const serverDate = clientDate.tz(serverTimezone).format('YYYY-MM-DDTHH:mm:ss');
```
2. **配置跨时区通信**:
如果你们有约定好的固定时区,比如总是使用UTC,确保在后端解析时也使用统一的时区。
3. **后端处理**:
同样地,确保后端在接收日期时能够正确处理这种时差,并转换回其内部标准。
在个人中心界面设置一个打卡按钮,每天只能打卡一次,直到另一天才能继续打卡,并将数据传到数据库,数据库有id,打卡用户id,打卡时间,第几天打卡的界面,写出springboot+vue代码
在Spring Boot项目中,我们可以使用Spring Data JPA结合Vue.js来实现这样的功能。首先,我们需要创建实体(Entity)、服务(Service)、Repository以及Vue页面。
**1. Entity (实体类) - UserCheckin.java**
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class UserCheckin {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Long userId; // 用户ID
private LocalDateTime checkinTime; // 打卡时间
private int day; // 当前打卡的天数
// getters and setters...
}
```
**2. Service (服务类) - CheckinService.java**
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.Date;
import java.time.LocalDateTime;
@Service
public class CheckinService {
@Autowired
private UserRepository userRepository;
public void dailyCheckin(Long userId) {
if (!isUserCheckedInToday(userId)) {
UserCheckin userCheckin = new UserCheckin();
userCheckin.setUserId(userId);
userCheckin.setDay(new Date().getDay() + 1); // 获取当前日期是一周中的第几天
userCheckin.setCheckinTime(LocalDateTime.now());
userRepository.save(userCheckin);
} else {
throw new IllegalStateException("今日已打卡");
}
}
private boolean isUserCheckedInToday(Long userId) {
return userRepository.existsByUserIdAndDay(userId, new Date().getDay() + 1);
}
}
```
**3. Repository (仓库接口) - UserRepository.java**
```java
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<UserCheckin, Long> {
boolean existsByUserIdAndDay(Long userId, int day);
}
```
**4. Vue 页面 (组件或路由文件)** - 在`/templates/daily-checkin.vue` 或者相应的模板文件中
```html
<template>
<button @click="checkin">今日打卡</button>
</template>
<script>
export default {
methods: {
checkin() {
axios.post('/api/checkin', { userId: this.userId }) // 假设userId存储在vuex里
.then(() => {
// 更新数据库状态(这里需要前端与后端交互)
// 如果有错误处理逻辑,可以添加异常捕获
})
.catch(error => console.error(error));
},
},
};
</script>
```
**
阅读全文
相关推荐
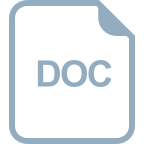
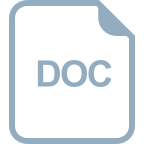
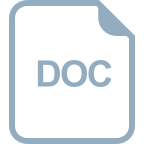
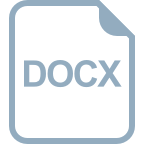
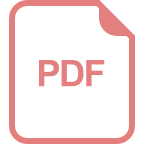
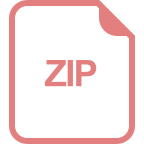
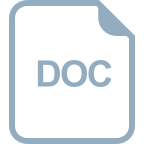
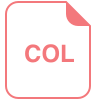
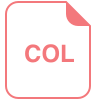
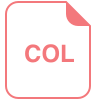
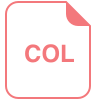
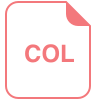
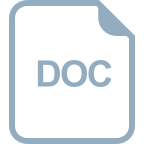
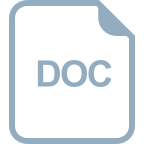