设计类似如下界面的功能,以实现图片道德打开,动态图的打开、播放一集停止。写出设计思路、设计步骤和编写的程序代码,
时间: 2024-04-16 19:24:15 浏览: 11
好的,根据您的要求,我可以提供一个设计思路和编程代码示例来实现您描述的界面和功能。
设计思路:
1. 创建一个界面,包含一个图片显示区域、一个按钮用于打开图片、一个按钮用于打开动态图、一个按钮用于播放/停止动态图。
2. 监听按钮的点击事件,根据不同的按钮执行相应的操作。
设计步骤:
1. 创建一个Qt应用程序,并设计界面。
2. 在界面中添加一个QLabel用于显示图片,以及三个QPushButton分别用于打开图片、打开动态图和播放/停止动态图。
3. 连接打开图片按钮的clicked信号到一个槽函数,槽函数中使用QFileDialog选择并加载图片,并在QLabel中显示。
4. 连接打开动态图按钮的clicked信号到一个槽函数,槽函数中使用QFileDialog选择并加载动态图,并在QLabel中显示第一帧。
5. 连接播放/停止动态图按钮的clicked信号到一个槽函数,槽函数中根据按钮状态播放或停止动态图。
编程代码示例(使用C++和Qt库):
```cpp
#include <QApplication>
#include <QMainWindow>
#include <QVBoxLayout>
#include <QLabel>
#include <QPushButton>
#include <QFileDialog>
#include <QMovie>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr) : QMainWindow(parent) {
QWidget *centralWidget = new QWidget();
QVBoxLayout *layout = new QVBoxLayout();
imageLabel = new QLabel();
openImageBtn = new QPushButton("打开图片");
openGifBtn = new QPushButton("打开动态图");
playStopBtn = new QPushButton("播放/停止动态图");
playStopBtn->setEnabled(false);
connect(openImageBtn, SIGNAL(clicked()), this, SLOT(openImage()));
connect(openGifBtn, SIGNAL(clicked()), this, SLOT(openGif()));
connect(playStopBtn, SIGNAL(clicked()), this, SLOT(playStopGif()));
layout->addWidget(imageLabel);
layout->addWidget(openImageBtn);
layout->addWidget(openGifBtn);
layout->addWidget(playStopBtn);
centralWidget->setLayout(layout);
setCentralWidget(centralWidget);
}
public slots:
void openImage() {
QString imagePath = QFileDialog::getOpenFileName(this, "选择图片", "", "Images (*.png *.xpm *.jpg)");
if (!imagePath.isEmpty()) {
QImage image(imagePath);
imageLabel->setPixmap(QPixmap::fromImage(image));
playStopBtn->setEnabled(false);
}
}
void openGif() {
QString gifPath = QFileDialog::getOpenFileName(this, "选择动态图", "", "GIF Images (*.gif)");
if (!gifPath.isEmpty()) {
movie = new QMovie(gifPath);
imageLabel->setMovie(movie);
playStopBtn->setEnabled(true);
}
}
void playStopGif() {
if (movie->state() == QMovie::Running) {
movie->stop();
playStopBtn->setText("播放动态图");
} else {
movie->start();
playStopBtn->setText("停止动态图");
}
}
private:
QLabel *imageLabel;
QPushButton *openImageBtn;
QPushButton *openGifBtn;
QPushButton *playStopBtn;
QMovie *movie;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MainWindow window;
window.show();
return app.exec();
}
```
以上是一个简单的示例,实现了图片的打开和显示,以及动态图的打开、播放和停止功能。您可以根据自己的需求进行修改和扩展。希望对您有帮助!
相关推荐
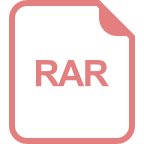
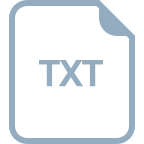
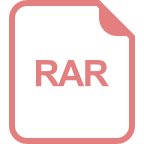














