使用RCLCPP编写节点,完成两个整数相加并输出结果的功能
时间: 2024-12-24 21:24:32 浏览: 6
在ROS 2 (Robot Operating System) 中,RCLcpp 是 C++ 版本的 ROS 接口库,它允许开发者创建、管理和服务节点(Node)。如果你想编写一个简单的 RCLcpp 节点来完成两个整数相加并输出结果,你可以按照以下步骤操作:
1. 首先,确保已安装了 ROS 2 和必要的开发工具包,如 `rclcpp` 和 `rcutils`。如果尚未安装,可以参考官方文档进行安装。
2. 创建一个新的 C++ 工程文件,例如 `add_two_integers.cpp`。在这个文件中,需要包含必要的头文件,并声明一个消息类型(如 `std_msgs::msg::Int32`),用于接收输入的整数。
```cpp
#include <rclcpp/rclcpp.hpp>
#include "std_msgs/msg/int32.hpp"
class AddTwoIntegers : public rclcpp::Node
{
public:
explicit AddTwoIntegers(rclcpp::NodeOptions options)
: Node("add_two_integers", options),
input_sub_(
this->create_subscription<std_msgs::msg::Int32>("input_topic", 10,
std::bind(&AddTwoIntegers::on_input_message, this, stdplaceholders::_1)))
{
}
private:
void on_input_message(const std_msgs::msg::Int32::SharedPtr msg)
{
int32_t first_number = msg->data;
rclcpp::Publisher<std_msgs::msg::Int32> output_pub = this->create_publisher<std_msgs::msg::Int32>("output_topic", 10);
int32_t result = first_number + another_number_; // 假设有一个全局变量another_number_
auto sum_msg = std_msgs::msg::Int32::make_shared();
sum_msg->data = result;
output_pub->publish(sum_msg);
RCLCPP_INFO(get_logger(), "Sum is %d", result);
}
rclcpp::Subscription<std_msgs::msg::Int32>::SharedPtr input_sub_;
};
```
3. 在 `main()` 函数中初始化节点,并启动消息循环:
```cpp
int main(int argc, char ** argv)
{
rclcpp::init(argc, argv);
AddTwoIntegers node;
rclcpp::spin(node); // 进入消息处理循环
rclcpp::shutdown(); // 关闭节点
return 0;
}
```
4. 编译这个节点,通过命令行运行它,并从终端发送两个整数到 `"input_topic"`,程序会计算它们的和并输出到 `"output_topic"`。
阅读全文
相关推荐
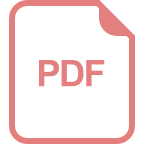
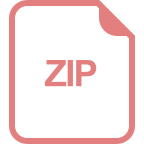
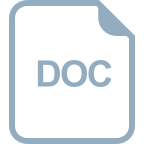
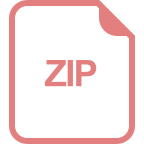
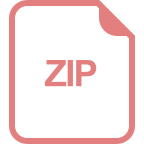
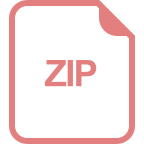
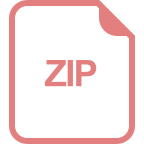
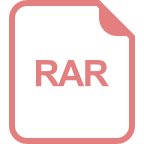
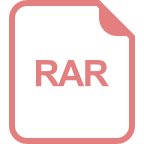
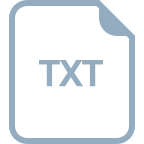
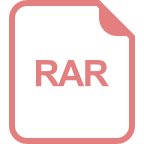
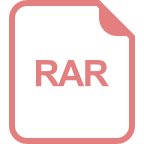
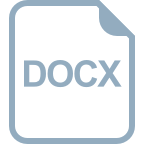
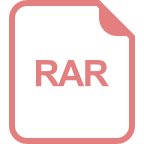
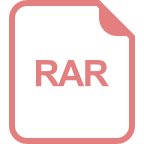
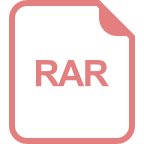
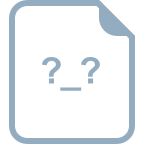
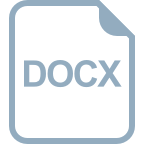