凯撒加密算法C++接收端和发送端代码
时间: 2024-10-13 22:05:40 浏览: 38
凯撒加密(Caesar Cipher),又称为移位密码,是一种古老的替换式加密技术。在C++中实现凯撒加密,通常涉及到对输入字符串中的每个字符按照固定步长进行移位。这里是简单的C++代码示例,包括发送端和接收端:
**发送端 (C++)**:
```cpp
#include <iostream>
#include <string>
std::string caesar_encrypt(const std::string& message, int shift) {
std::string encrypted;
for (char c : message) {
if (isalpha(c)) { // 检查是否为字母
char shifted = static_cast<char>((c + shift - 'A') % 26 + 'A'); // 移动并保持大写字母范围
if (islower(c))
shifted = static_cast<char>((c + shift - 'a') % 26 + 'a'); // 对小写字母同样处理
encrypted += shifted;
} else {
encrypted += c; // 非字母字符不变
}
}
return encrypted;
}
int main() {
std::string original = "Hello, World!";
int shift_amount = 3;
std::string encrypted_text = caesar_encrypt(original, shift_amount);
std::cout << "Original: " << original << "\n";
std::cout << "Encrypted: " << encrypted_text << "\n";
return 0;
}
```
**接收端 (C++)**:
由于接收端需要逆向进行解密,只需将上面的`caesar_encrypt`函数稍微修改一下:
```cpp
std::string caesar_decrypt(const std::string& encrypted, int shift) {
return caesar_encrypt(encrypted, -shift); // 使用相反的移位量进行解密
}
// 在主函数里解密...
```
**相关问题--:**
1. 发送端和接收端如何交互数据以便进行加密和解密?
2. 在实际应用中,凯撒加密有哪些局限性?
3. 如何增加凯撒加密的安全性?
阅读全文
相关推荐
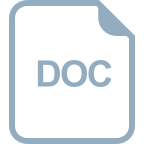
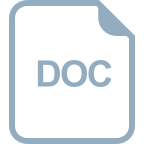
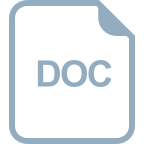
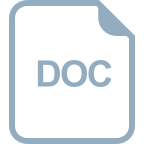
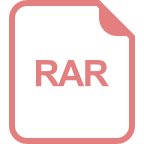
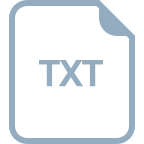





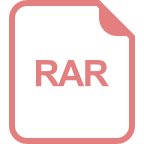
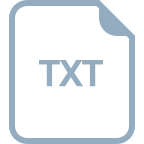
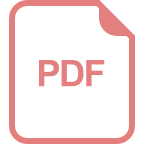
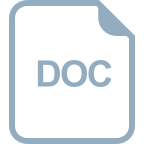

