uniapp vue锚点
时间: 2024-01-23 22:15:23 浏览: 26
在uniapp中,可以使用vue的方法来实现锚点定位。具体步骤如下:
1. 在需要跳转到的目标位置添加一个id属性,例如:
```html
<div id="target"></div>
```
2. 在触发跳转的位置添加一个点击事件,并在事件处理函数中使用`document.querySelector().scrollIntoView()`方法来实现锚点定位,例如:
```html
<template>
<div>
<button @click="scrollToTarget">跳转到目标位置</button>
</div>
</template>
<script>
export default {
methods: {
scrollToTarget() {
document.querySelector('#target').scrollIntoView(true);
}
}
}
</script>
```
这样,当点击按钮时,页面会自动滚动到目标位置。
相关问题
uniapp vue3
你好!关于 UniApp 和 Vue 3 的结合,以下是一些常见的问题和答案:
1. UniApp 支持 Vue 3 吗?
目前,UniApp 尚未全面支持 Vue 3。UniApp 目前仍然基于 Vue 2.x 版本进行开发。但是,UniApp 官方已经在考虑和测试支持 Vue 3,未来可能会有相应的升级。
2. 如何在 UniApp 中使用 Vue 3 的新特性?
目前在 UniApp 中使用 Vue 3 的新特性可能会遇到一些限制。但是,你仍然可以使用一些 Vue 3 的特性,比如 Composition API,通过使用 `@vue/composition-api` 插件来实现。
3. 我应该继续使用 Vue 2.x 还是等待 UniApp 对 Vue 3 的支持?
这个取决于你的项目需求和时间安排。如果你的项目紧急,并且你不想等待或者无法等待 UniApp 对 Vue 3 的支持,那么继续使用 Vue 2.x 是一个不错的选择。如果你可以等待并且希望使用 Vue 3 的新特性,可以关注 UniApp 官方的更新和升级。
总结起来,目前 UniApp 尚未全面支持 Vue 3,但你仍然可以在 UniApp 中使用一些 Vue 3 的特性。如果你对 Vue 3 特性有强烈需求,你可以等待 UniApp 对 Vue 3 的支持,或者考虑其他支持 Vue 3 的框架。希望这些信息对你有帮助!如果还有其他问题,请随时提问。
uniapp vue2
UniApp是一款基于Vue.js的跨平台开发框架,它可以同时开发iOS、Android、H5等多个平台的应用程序。UniApp使用了Vue.js的语法和组件化开发思想,使得开发者可以用一套代码编写多个平台的应用,大大提高了开发效率。
UniApp的核心是基于Vue.js的组件化开发,开发者可以使用Vue.js的语法进行页面的开发和数据的绑定。同时,UniApp还提供了一些特定平台的API和组件,可以方便地调用原生功能和实现特定平台的交互效果。
UniApp支持使用Vue.js的全套特性,包括响应式数据绑定、组件化开发、路由管理、状态管理等。开发者可以使用Vue.js的生态系统中的插件和工具来辅助开发,如Vuex、Vue Router等。
UniApp还提供了一套丰富的UI组件库,可以快速构建各种样式的界面。同时,UniApp还支持使用原生小程序组件和自定义组件,可以满足更复杂的界面需求。
总结一下,UniApp是一款基于Vue.js的跨平台开发框架,它可以帮助开发者用一套代码编写多个平台的应用程序,提高开发效率和代码复用性。
相关推荐
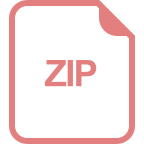












