蚁群算法之旅行商问题(TSP)
时间: 2024-12-27 07:30:18 浏览: 4
### 蚁群算法解决TSP旅行商问题
#### 算法原理
蚁群优化算法是一种基于自然现象——蚂蚁觅食行为的元启发式算法。该方法模仿真实世界中的蚂蚁群体,它们通过释放一种称为信息素的化学物质来标记路径,从而影响其他蚂蚁的选择倾向。在求解TSP时,每只虚拟蚂蚁构建一个完整的环游路线,并依据当前节点到下一个访问城市的距离以及连接这两点间残留的信息素浓度决定移动方向。
#### 参数设置
为了有效应用蚁群算法于TSP实例中,需定义四个主要参数:
- **α (Alpha)**:表示信息素重要性的权重系数。
- **β (Beta)** :代表启发函数的重要性程度。
- **ρ (Rho)** :蒸发率,用于模拟自然界里随着时间推移信息素逐渐消失的过程。
- **Q** :常量,用来更新路径上的新增信息素总量[^1]。
这些因素共同作用决定了模型性能的好坏,在实际操作过程中可根据具体情况进行调整以获得更好的效果。
#### 实现过程
下面给出一段简单的 Python 代码片段展示如何实现基本版的 ACO 来处理 TSP:
```python
import numpy as np
from random import randint, uniform
def initialize_ants(num_cities):
ants = []
for _ in range(num_cities):
start_city = randint(0, num_cities - 1)
ant_path = [start_city]
visited = set([start_city])
while len(visited) != num_cities:
next_city = select_next_city(start_city, visited)
ant_path.append(next_city)
visited.add(next_city)
ants.append((ant_path))
return ants
def update_pheromones(paths, distances, pheromone_matrix, Q=100, rho=.5):
n = len(distances)
delta_tau = [[0]*n for i in range(n)]
for path in paths:
total_distance = sum(distances[path[i]][path[(i+1)%len(path)]] for i in range(len(path)))
try:
contribution = Q / total_distance
except ZeroDivisionError:
continue
for i in range(len(path)):
current = path[i]
next_node = path[(i + 1) % len(path)]
delta_tau[current][next_node] += contribution
new_pheromone_matrix = [(1-rho)*pheromone_matrix[i][j]+delta_tau[i][j]
for i in range(n) for j in range(n)]
return np.array(new_pheromone_matrix).reshape((n,n))
# Note: The function `select_next_city` and other helper functions are not fully implemented here.
```
此段程序展示了初始化蚂蚁种群、计算并更新信息素矩阵的核心逻辑。需要注意的是,这里省略了一些辅助功能的具体实现细节,比如选择下一座城市的方法等。完整版本还需要加入更多机制来提高效率和准确性[^2]。
阅读全文
相关推荐
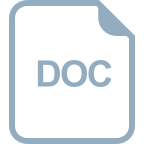
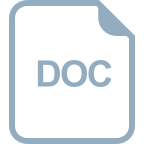
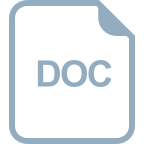
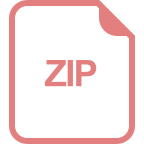
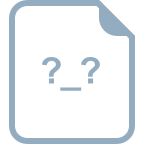
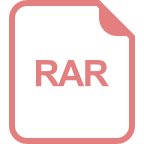
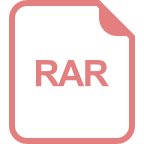
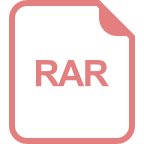
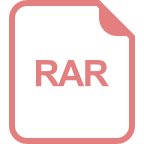
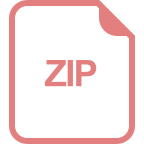
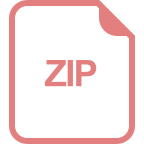
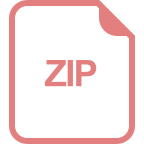
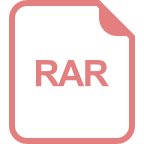
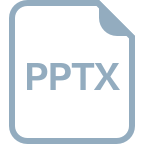
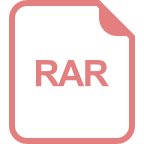
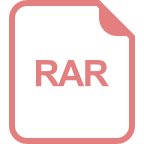
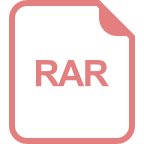