c语言怎么从文件中读取数据到链表
时间: 2024-06-14 17:04:51 浏览: 116
以下是使用C语言从文件中读取数据到链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 读取文件数据到链表
struct Node* readFileToList(const char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file.\n");
return NULL;
}
struct Node* head = NULL;
struct Node* tail = NULL;
int value;
// 逐行读取文件数据
while (fscanf(file, "%d", &value) != EOF) {
// 创建新节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
// 将节点插入链表尾部
if (head == NULL) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
fclose(file);
return head;
}
// 打印链表数据
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
const char* filename = "data.txt";
struct Node* head = readFileToList(filename);
if (head != NULL) {
printf("Data in the list: ");
printList(head);
}
return 0;
}
```
阅读全文
相关推荐
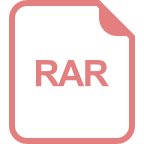
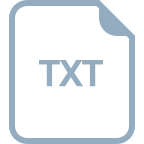
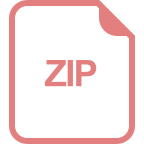
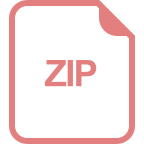
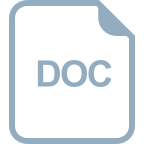
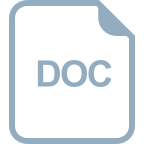
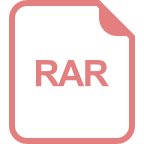
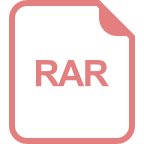
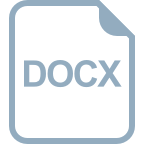
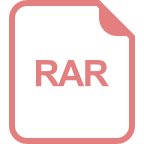
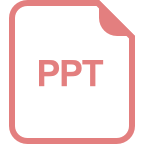
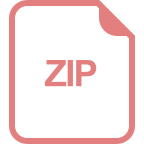
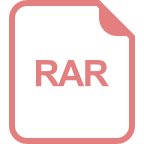
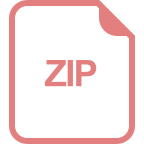