编写程序,创建两个线程,分别输出10个100以内的随机正整数,每输出一个整数随机休眠几秒钟。
时间: 2023-09-21 17:03:08 浏览: 82
以下是Python代码实现:
```python
import threading
import random
import time
def thread_func():
for i in range(10):
num = random.randint(1, 100)
print(threading.current_thread().name, "generated", num)
time.sleep(random.random())
t1 = threading.Thread(target=thread_func)
t2 = threading.Thread(target=thread_func)
t1.start()
t2.start()
t1.join()
t2.join()
```
代码解释:
1. `threading` 模块用于创建和管理线程。
2. `random` 模块用于生成随机数。
3. `time` 模块用于休眠一定时间。
4. `thread_func` 函数是线程的执行函数,用于生成随机数并输出。
5. `t1` 和 `t2` 是两个线程对象,分别调用 `thread_func` 函数。
6. `start` 方法用于启动线程。
7. `join` 方法用于等待线程结束。
8. `threading.current_thread().name` 返回当前线程的名称,用于区分两个线程输出的随机数。
相关问题
编写程序,创建两个线程,分别输出10个100以内的随机正整数,每输出一个整数随机休眠几秒钟。python
import threading
import random
import time
def print_numbers(id):
print(f"Thread {id} starts printing numbers:")
for i in range(10):
num = random.randint(1, 100)
print(f"Thread {id}: {num}")
time.sleep(random.uniform(0.5, 2.0)) # random sleep time between 0.5 and 2.0 seconds
# create two threads
t1 = threading.Thread(target=print_numbers, args=(1,))
t2 = threading.Thread(target=print_numbers, args=(2,))
# start the threads
t1.start()
t2.start()
# wait for the threads to finish
t1.join()
t2.join()
print("All threads have finished.")
编写python程序,创建两个线程,分别输出10个100以内的随机正整数,每输出一个整数随机休眠几秒钟。
下面是一个实现该功能的Python程序:
```python
import threading
import random
import time
# 定义一个输出随机整数的函数
def print_random_int():
for i in range(10):
print(random.randint(1, 100))
time.sleep(random.random()*3) # 随机休眠几秒钟
# 创建两个线程并启动
t1 = threading.Thread(target=print_random_int)
t2 = threading.Thread(target=print_random_int)
t1.start()
t2.start()
# 等待两个线程执行完毕
t1.join()
t2.join()
```
该程序首先定义了一个`print_random_int()`函数,用于输出10个随机整数并随机休眠几秒钟。然后创建了两个线程`t1`和`t2`,分别指向该函数,并启动这两个线程。最后使用`join()`方法等待两个线程执行完毕。
在执行该程序时,会输出两个线程各自的10个随机整数,每个整数后面会随机休眠几秒钟。由于随机休眠时间的存在,每次运行结果都会有所不同。
阅读全文
相关推荐









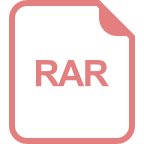
