jupyter统计自己分词后的词频
时间: 2023-07-23 11:10:43 浏览: 101
在Jupyter中,可以使用相同的代码来统计自己分词后的词频。需要先在Jupyter中安装jieba和Counter模块,可以使用以下命令:
```
!pip install jieba
```
然后,在Jupyter中执行以下代码:
```python
from collections import Counter
import jieba
text = "这是一个测试文本,测试文本用来测试分词器的效果。"
word_list = jieba.lcut(text)
word_count = Counter(word_list)
print(word_count)
```
执行结果将会输出单词及其对应的出现次数。
相关问题
使用jupyter notebook进行程序编写1.使用Pandas读取数据集。 2.统计每年的发文数量,并绘制折线图。 3.统计出版社的发文量信息,列出发文前10位的出版社。 4.使用jieba分词,对摘要进行分词统计,制作词频前30位的词
好的,让我们一步步来实现这些功能。
首先,我们需要安装必要的库,包括pandas, matplotlib和jieba。你可以在命令行中使用以下命令进行安装。
```
pip install pandas matplotlib jieba
```
接下来,我们将使用pandas来读取数据集。假设数据集名为"articles.csv",并位于当前工作目录中。我们可以使用以下代码读取它。
```python
import pandas as pd
df = pd.read_csv('articles.csv')
```
现在,我们已经成功加载了数据集。接下来,我们将使用pandas和matplotlib来统计每年的发文数量,并绘制折线图。
```python
import matplotlib.pyplot as plt
# 添加一列"year",用于存储每篇文章的年份
df['year'] = pd.DatetimeIndex(df['publish_time']).year
# 统计每个年份的文章数量
year_counts = df['year'].value_counts().sort_index()
# 绘制折线图
plt.plot(year_counts.index, year_counts.values)
plt.xlabel('Year')
plt.ylabel('Number of Articles')
plt.show()
```
现在,我们已经成功绘制了每年的发文数量折线图。接下来,我们将统计出版社的发文量信息,并列出前10位的出版社。
```python
# 统计每个出版社的文章数量
publisher_counts = df['journal'].value_counts()
# 列出前10位的出版社
top_publishers = publisher_counts[:10]
print(top_publishers)
```
我们可以看到前10位的出版社及其文章数量。现在,我们将使用jieba来分词并统计摘要中的词频。
```python
import jieba
from collections import Counter
# 定义分词函数
def cut_words(text):
words = jieba.cut(text)
return [word for word in words if len(word) > 1]
# 对每篇文章的摘要进行分词
abstracts = df['abstract'].dropna().apply(cut_words)
# 合并所有摘要的分词结果
all_words = [word for abstract in abstracts for word in abstract]
# 统计词频并列出前30位
word_counts = Counter(all_words)
top_words = word_counts.most_common(30)
print(top_words)
```
现在,我们已经成功地使用了pandas,matplotlib和jieba来完成了这些任务。
使用jupyter notebook进行程序编写1.使用Pandas读取数据集。 2.统计每年的发文数量,并绘制折线图。 3.统计出版社的发文量信息,列出发文前10位的出版社。 4.使用jieba分词,对摘要进行分词统计,制作词频前30位的词云图。(需安装jieba分词和词云工具包)。
好的,让我们逐步实现这些步骤。
首先,我们需要安装必要的库。打开命令行窗口,输入以下命令:
```python
pip install pandas matplotlib jieba wordcloud
```
这将安装所需的库。
接下来,我们可以开始编写代码。首先,我们需要导入所需的库:
```python
import pandas as pd
import matplotlib.pyplot as plt
import jieba
from wordcloud import WordCloud
```
然后,我们可以使用Pandas读取数据集。假设数据集是一个CSV文件,名为“articles.csv”,并且位于当前工作目录中。我们可以使用以下代码读取数据集:
```python
df = pd.read_csv('articles.csv')
```
接下来,我们可以统计每年的发文数量,并绘制折线图。我们可以使用Pandas中的groupby和count函数来实现这一点,然后使用Matplotlib库来绘制折线图。
```python
# 统计每年的发文数量
yearly_counts = df.groupby(df['year']).count()['title']
# 绘制折线图
plt.plot(yearly_counts.index, yearly_counts.values)
plt.xlabel('Year')
plt.ylabel('Number of Articles')
plt.show()
```
接下来,我们可以统计出版社的发文量信息,并列出发文前10位的出版社。我们可以使用Pandas中的groupby和count函数来实现这一点。
```python
# 统计出版社的发文量信息
publisher_counts = df.groupby(df['publisher']).count()['title']
# 列出发文前10位的出版社
top_publishers = publisher_counts.sort_values(ascending=False)[:10]
print(top_publishers)
```
最后,我们可以使用jieba分词,对摘要进行分词统计,制作词频前30位的词云图。我们可以使用jieba和WordCloud库来实现这一点。
```python
# 对摘要进行分词
abstracts = ' '.join(df['abstract'])
words = jieba.cut(abstracts)
# 统计词频
word_counts = {}
for word in words:
if len(word) > 1:
word_counts[word] = word_counts.get(word, 0) + 1
# 制作词云图
wc = WordCloud(width=800, height=400, background_color='white', max_words=30)
wc.generate_from_frequencies(word_counts)
plt.imshow(wc)
plt.axis('off')
plt.show()
```
这就是我们的完整代码。注意,以上代码只是示例代码,具体实现方式可能会因数据集的不同而有所变化。
阅读全文
相关推荐
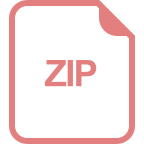
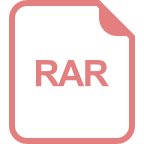
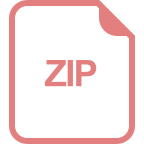
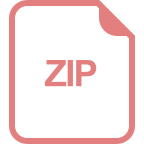
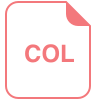
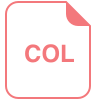
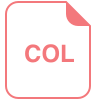
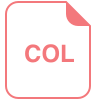




