stm32 18b20 代码
时间: 2023-07-02 16:18:07 浏览: 32
以下是使用STM32和DS18B20温度传感器进行温度测量的示例代码:
```c
#include "stm32f4xx.h"
#define DQ GPIO_Pin_0 //定义DQ为GPIO_Pin_0
void delay_us(uint32_t us)
{
us *= 12;
while(us--)
__NOP();
}
void delay_ms(uint32_t ms)
{
while(ms--)
delay_us(1000);
}
void DS18B20_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = DQ;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_OD;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_SetBits(GPIOA, DQ);
}
void DS18B20_Reset(void)
{
GPIO_ResetBits(GPIOA, DQ);
delay_us(480);
GPIO_SetBits(GPIOA, DQ);
delay_us(60);
}
uint8_t DS18B20_ReadBit(void)
{
uint8_t data;
GPIO_ResetBits(GPIOA, DQ);
delay_us(2);
GPIO_SetBits(GPIOA, DQ);
delay_us(10);
data = GPIO_ReadInputDataBit(GPIOA, DQ);
delay_us(50);
return data;
}
uint8_t DS18B20_ReadByte(void)
{
uint8_t i, j, data = 0;
for(i=0; i<8; i++)
{
j = DS18B20_ReadBit();
data |= (j<<i);
}
return data;
}
void DS18B20_WriteBit(uint8_t data)
{
GPIO_ResetBits(GPIOA, DQ);
delay_us(2);
if(data)
GPIO_SetBits(GPIOA, DQ);
else
GPIO_ResetBits(GPIOA, DQ);
delay_us(60);
GPIO_SetBits(GPIOA, DQ);
}
void DS18B20_WriteByte(uint8_t data)
{
uint8_t i;
for(i=0; i<8; i++)
{
DS18B20_WriteBit(data & 0x01);
data >>= 1;
}
}
float DS18B20_GetTemp(void)
{
uint8_t tempL, tempH;
float temp;
DS18B20_Reset();
DS18B20_WriteByte(0xcc);
DS18B20_WriteByte(0x44);
delay_ms(750);
DS18B20_Reset();
DS18B20_WriteByte(0xcc);
DS18B20_WriteByte(0xbe);
tempL = DS18B20_ReadByte();
tempH = DS18B20_ReadByte();
temp = (tempH<<8) | tempL;
temp *= 0.0625;
return temp;
}
int main(void)
{
float temp;
DS18B20_Init();
while(1)
{
temp = DS18B20_GetTemp();
/* 处理温度值 */
}
}
```
这段代码实现了DS18B20温度传感器的初始化、复位、读取温度等操作。需要注意的是,该代码使用了GPIOA的第0位作为DS18B20的数据线。在使用时,需要将代码中的GPIO引脚定义修改为实际使用的引脚。
相关推荐
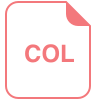
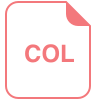
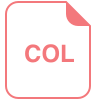
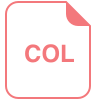
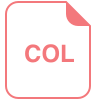









