双向链表插入节点python
时间: 2023-06-28 13:08:47 浏览: 43
以下是一个简单的 Python 双向链表插入节点的示例代码:
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
self.prev = None
class DoublyLinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if self.head is None:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
new_node.prev = current
def insert(self, data, position):
new_node = Node(data)
current = self.head
if position == 0:
new_node.next = current
current.prev = new_node
self.head = new_node
else:
for i in range(position-1):
current = current.next
new_node.next = current.next
new_node.prev = current
if current.next:
current.next.prev = new_node
current.next = new_node
def print_list(self):
current = self.head
while current:
print(current.data)
current = current.next
# Example usage
linked_list = DoublyLinkedList()
linked_list.append(1)
linked_list.append(2)
linked_list.append(4)
# Insert node with value 3 at index 2 (0-based indexing)
linked_list.insert(3, 2)
# Print the resulting list
linked_list.print_list()
```
运行结果如下:
```
1
2
3
4
```
相关推荐
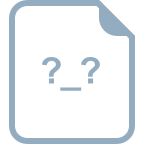
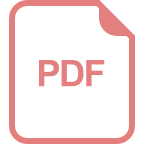















