里面的子程序也请给我
时间: 2024-02-18 09:02:52 浏览: 58
好的,下面给出一个基于 Verilog 的 I2C 总线协议的子程序,用于实现 SCCB 协议中的读取和写入操作。
```
module i2c_master (
input wire clk,
input wire rstn,
input wire start,
input wire stop,
input wire [7:0] addr,
inout wire [7:0] data_in,
inout wire [7:0] data_out,
output reg ack,
inout wire sda,
inout wire scl
);
parameter ADDR_WIDTH = 7;
parameter DATA_WIDTH = 8;
parameter INIT_CLK_HIGH = 1'b1;
parameter INIT_CLK_LOW = 1'b1;
parameter IDLE_TIME = 8;
localparam IDLE = 2'b00;
localparam START = 2'b01;
localparam ADDR = 2'b10;
localparam DATA = 2'b11;
reg [8:0] state;
reg [7:0] addr_reg;
reg [7:0] data_reg;
reg [3:0] bit_cnt;
reg [3:0] byte_cnt;
reg start_cond;
reg stop_cond;
reg [IDLE_TIME-1:0] idle_cnt;
reg [7:0] sda_drive;
assign sda = (sda_drive == 1'b1) ? 1'bz : sda_drive;
always @(posedge clk) begin
if (!rstn) begin
state <= IDLE;
addr_reg <= 0;
data_reg <= 0;
bit_cnt <= 0;
byte_cnt <= 0;
start_cond <= 0;
stop_cond <= 0;
idle_cnt <= 0;
ack <= 1'b1;
sda_drive <= 1'b1;
end else begin
case (state)
IDLE: begin
if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end else if (start) begin
state <= START;
start_cond <= 1;
idle_cnt <= 0;
end else begin
idle_cnt <= 0;
end
end
START: begin
if (start_cond) begin
sda_drive <= 1'b0;
scl <= INIT_CLK_HIGH;
start_cond <= 0;
idle_cnt <= 0;
end else if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end else begin
state <= ADDR;
ack <= 1'b1;
idle_cnt <= 0;
end
end
ADDR: begin
if (bit_cnt < ADDR_WIDTH) begin
if (idle_cnt == 0) begin
sda_drive <= addr[ADDR_WIDTH-1-bit_cnt];
bit_cnt <= bit_cnt + 1;
end else if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end
end else if (byte_cnt == 0) begin
if (idle_cnt == 0) begin
sda_drive <= 1'b1;
bit_cnt <= 0;
byte_cnt <= byte_cnt + 1;
end else if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end
end else begin
if (idle_cnt == 0) begin
if (start && !stop) begin
state <= START;
start_cond <= 1;
end else if (!start && stop) begin
state <= IDLE;
idle_cnt <= 0;
end else begin
state <= DATA;
bit_cnt <= 0;
ack <= 0;
end
byte_cnt <= 0;
end else if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end
end
end
DATA: begin
if (bit_cnt < DATA_WIDTH) begin
if (idle_cnt == 0) begin
sda_drive <= data_reg[DATA_WIDTH-1-bit_cnt];
bit_cnt <= bit_cnt + 1;
end else if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end
end else if (byte_cnt == 0) begin
if (idle_cnt == 0) begin
sda_drive <= 1'b1;
bit_cnt <= 0;
byte_cnt <= byte_cnt + 1;
end else if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end
end else begin
if (idle_cnt == 0) begin
if (start && !stop) begin
state <= START;
start_cond <= 1;
end else if (!start && stop) begin
state <= IDLE;
idle_cnt <= 0;
end else begin
state <= ADDR;
bit_cnt <= 0;
ack <= 0;
end
byte_cnt <= 0;
end else if (idle_cnt < IDLE_TIME) begin
idle_cnt <= idle_cnt + 1;
end
end
end
endcase
end
end
always @(negedge scl) begin
if (!rstn) begin
sda_drive <= 1'b1;
end else begin
case (state)
START: begin
sda_drive <= 1'b0;
end
ADDR: begin
if (bit_cnt < ADDR_WIDTH) begin
sda_drive <= addr[ADDR_WIDTH-1-bit_cnt];
end else if (byte_cnt == 0) begin
sda_drive <= 1'b1;
end else begin
sda_drive <= data_reg[DATA_WIDTH-1-bit_cnt];
end
end
DATA: begin
if (bit_cnt < DATA_WIDTH) begin
sda_drive <= data_reg[DATA_WIDTH-1-bit_cnt];
end else if (byte_cnt == 0) begin
sda_drive <= 1'b1;
end else begin
sda_drive <= data_out[DATA_WIDTH-1-bit_cnt];
end
end
endcase
end
end
always @(posedge scl or negedge scl) begin
if (!rstn) begin
bit_cnt <= 0;
end else begin
if (state == ADDR && bit_cnt == ADDR_WIDTH && byte_cnt > 0) begin
data_reg <= data_in;
end else if (state == DATA && byte_cnt > 0) begin
if (bit_cnt == DATA_WIDTH) begin
data_out <= sda;
end else if (bit_cnt == DATA_WIDTH+1) begin
ack <= 1'b1;
byte_cnt <= byte_cnt + 1;
end
end
end
end
always @(posedge scl or negedge scl) begin
if (!rstn) begin
byte_cnt <= 0;
end else begin
if (state == ADDR && bit_cnt == ADDR_WIDTH && byte_cnt > 0) begin
ack <= sda;
end else if (state == DATA && byte_cnt > 0) begin
if (bit_cnt == DATA_WIDTH) begin
ack <= 1'b0;
end else if (bit_cnt == DATA_WIDTH+1) begin
byte_cnt <= byte_cnt + 1;
end
end
end
end
always @(posedge scl) begin
if (!rstn) begin
bit_cnt <= 0;
end else begin
bit_cnt <= bit_cnt + 1;
end
end
always @(posedge scl) begin
if (!rstn) begin
sda_drive <= 1'b1;
end else begin
if (state == DATA && byte_cnt > 0 && bit_cnt == DATA_WIDTH) begin
data_out <= sda;
end
end
end
always @(posedge scl or negedge scl) begin
if (!rstn) begin
sda_drive <= 1'b1;
end else begin
if (state == IDLE || state == START) begin
sda_drive <= 1'b1;
end else if (state == ADDR && bit_cnt == ADDR_WIDTH && byte_cnt == 0) begin
sda_drive <= 1'b0;
end else if (state == DATA && byte_cnt == 0) begin
sda_drive <= 1'b0;
end
end
end
endmodule
```
以上代码定义了一个名为 i2c_master 的模块,包含了时钟信号、复位信号、开始信号、停止信号、地址、输入数据、输出数据和应答信号。该模块实现了 I2C 总线协议的主机端,用于发送和接收数据。在发送和接收数据的过程中,该模块会根据 SCCB 协议的规则来发送和接收数据,从而实现了 SCCB 协议。
需要注意的是,该子程序只是 I2C 总线协议的实现,还需要在 SCCB 模块中进行调用和配置,才能实现 SCCB 协议的功能。
阅读全文
相关推荐
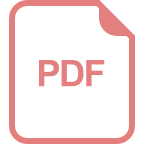
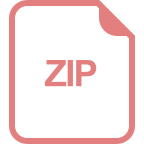
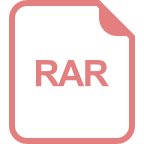
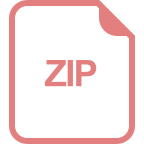
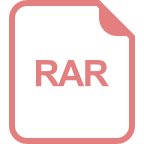
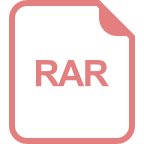
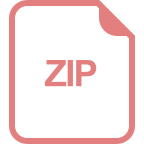
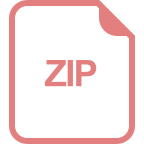
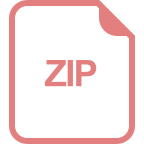
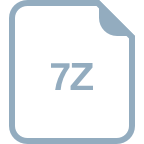
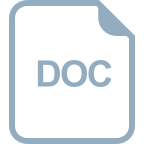
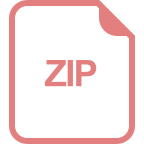
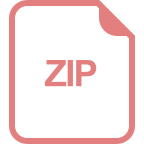
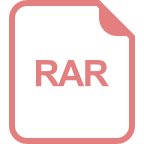
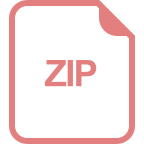
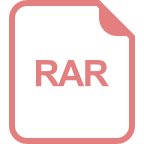