Extend the application above to be able to measure the efficiency of the implemented sorting algorithm in term of number of comparisons performed by the algorithm. The application should display the total number of comparisons performed by the algorithm for a given collection of items. [10 marks]
时间: 2023-08-21 17:23:55 浏览: 106
Here is an updated implementation of the NetBeans application that includes the ability to measure the efficiency of the sorting algorithm in terms of the number of comparisons performed:
```java
import java.util.ArrayList;
public class MyArrayList<T extends Comparable<T>> extends ArrayList<T> {
private int numComparisons;
public void bubbleSortDescending() {
int n = this.size();
boolean swapped;
do {
swapped = false;
for (int i = 1; i < n; i++) {
if (this.get(i - 1).compareTo(this.get(i)) < 0) {
T temp = this.get(i - 1);
this.set(i - 1, this.get(i));
this.set(i, temp);
swapped = true;
}
numComparisons++;
}
n--;
} while (swapped);
}
public int getNumComparisons() {
return numComparisons;
}
public static void main(String[] args) {
MyArrayList<Integer> list = new MyArrayList<Integer>();
list.add(7);
list.add(2);
list.add(9);
list.add(4);
list.add(5);
list.add(1);
list.add(6);
list.add(8);
list.add(3);
list.add(10);
System.out.println("Original list: " + list);
list.bubbleSortDescending();
System.out.println("Sorted list: " + list);
System.out.println("Number of comparisons: " + list.getNumComparisons());
}
}
```
In this updated implementation, we add a private instance variable `numComparisons` to keep track of the number of comparisons performed by the sorting algorithm. We increment this variable every time a comparison is made in the `bubbleSortDescending` method.
We also add a getter method `getNumComparisons` to retrieve the value of `numComparisons` from outside the class.
In the `main` method, after sorting the list, we print out the number of comparisons performed by the algorithm using the `getNumComparisons` method.
阅读全文
相关推荐
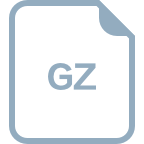

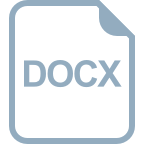
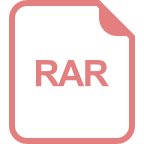
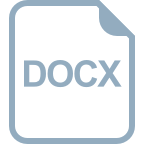
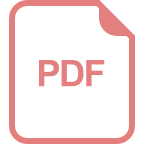
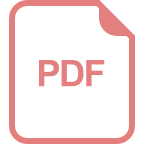
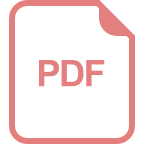
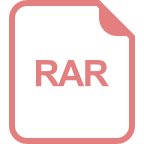
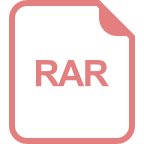
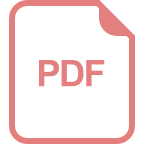
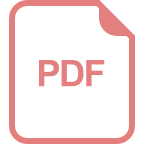
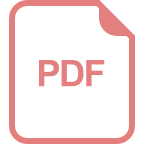
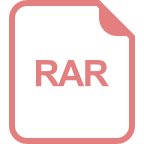