C++QtTableView最后一行改颜色
时间: 2024-12-19 14:24:38 浏览: 6
在C++ Qt中,如果你想要改变QTableView的最后一行的颜色,你可以通过动态创建模型数据并在`paintEvent()`函数中处理样式来实现。这里是一个简单的步骤:
1. 首先,确保你在`QAbstractItemModel`派生类(如`QStandardItemModel`或自定义的`MyTableModel`)中设置了正确的列数和行数。
```cpp
class MyTableModel : public QStandardItemModel {
public:
// 添加一行到模型末尾
void addLastRow(const QModelIndex &parent = QModelIndex()) override {
// ...
emit dataChanged(parent, parent); // 更新视图
}
};
```
2. 在`MyTableModel`中添加一个标志,用于标记是否是最后一行:
```cpp
bool MyTableModel::isLastRow(const QModelIndex &index) const {
if (index.row() == rowCount() - 1) {
return true;
}
return false;
}
```
3. 然后,在`paintEvent(QPaintEvent *event)`中检查当前绘制的行是否是最后一行,并应用特殊的背景色:
```cpp
void MyTableModel::paintRow(QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const {
if (isLastRow(index)) {
option.palette.setColor(QPalette::Background, yourCustomColor);
}
// 继续正常的画线逻辑...
QApplication::style()->drawControl(QStyle::CE_ItemViewItem, &option, painter, this);
}
```
4. 当插入或删除行时,确保更新视图以便样式生效:
```cpp
void MyTableModel::insertRows(int row, int count, const QModelIndex &parent = QModelIndex()) {
// ...
for (int i = 0; i < count; ++i) {
addLastRow(parent.child(row + i));
}
emit dataChanged(parent, parent.bottom());
}
void MyTableModel::removeRows(int row, int count, const QModelIndex &parent = QModelIndex()) {
// ...
for (int i = 0; i < count; ++i) {
if (isLastRow(parent.child(row + i))) {
option.palette.setColor(QPalette::Background, Qt::white); // 恢复默认背景色
}
}
emit dataChanged(parent, parent.bottom());
}
```
阅读全文
相关推荐
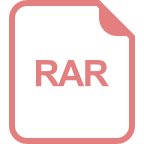
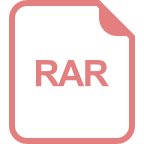
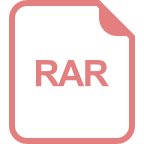



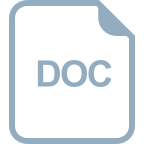


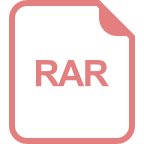
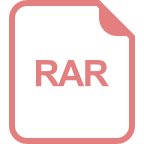
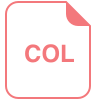







