如何在katalonstudio中添加自动化比较文件的代码
时间: 2023-11-28 15:49:10 浏览: 84
在Katalon Studio中,可以使用以下代码来进行自动化比较文件:
```java
import com.kms.katalon.core.util.KeywordUtil;
import com.kms.katalon.core.testobject.ConditionType;
import com.kms.katalon.core.testobject.TestObject;
import com.kms.katalon.core.testobject.TestObjectProperty;
import com.kms.katalon.core.testobject.TestObjectPropertyBuilder;
import com.kms.katalon.core.testobject.TestObjectPropertyType;
import com.kms.katalon.core.testobject.TestObjectPropertyStrategy;
import org.apache.commons.io.FileUtils;
import org.junit.Assert;
import java.io.File;
import java.io.IOException;
public class FileCompare {
/**
* Compare two files.
*
* @param file1
* @param file2
* @return true if both files are same otherwise false
*/
public static boolean compareFiles(File file1, File file2) {
try {
return FileUtils.contentEquals(file1, file2);
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
/**
* Compare two files by their paths.
*
* @param filePath1
* @param filePath2
* @return true if both files are same otherwise false
*/
public static boolean compareFiles(String filePath1, String filePath2) {
File file1 = new File(filePath1);
File file2 = new File(filePath2);
return compareFiles(file1, file2);
}
/**
* Verify two files are same or not.
*
* @param file1
* @param file2
* @param message
*/
public static void verifyFiles(File file1, File file2, String message) {
boolean result = compareFiles(file1, file2);
Assert.assertTrue(message, result);
}
/**
* Verify two files are same or not.
*
* @param filePath1
* @param filePath2
* @param message
*/
public static void verifyFiles(String filePath1, String filePath2, String message) {
boolean result = compareFiles(filePath1, filePath2);
Assert.assertTrue(message, result);
}
/**
* Verify two files are same or not.
*
* @param testObject1
* @param testObject2
* @param message
*/
public static void verifyFiles(TestObject testObject1, TestObject testObject2, String message) {
File file1 = new File(testObject1.getFilePath());
File file2 = new File(testObject2.getFilePath());
verifyFiles(file1, file2, message);
}
/**
* Verify two files are same or not.
*
* @param testObjectName1
* @param testObjectName2
* @param message
*/
public static void verifyFiles(String testObjectName1, String testObjectName2, String message) {
TestObject testObject1 = new TestObject(testObjectName1);
TestObjectPropertyBuilder builder1 = new TestObjectPropertyBuilder()
.withName(testObjectName1)
.withType(TestObjectPropertyType.FILE_TYPE)
.withStrategy(TestObjectPropertyStrategy.OPTIONAL);
TestObjectProperty property1 = builder1.build();
testObject1.addProperty(property1);
TestObject testObject2 = new TestObject(testObjectName2);
TestObjectPropertyBuilder builder2 = new TestObjectPropertyBuilder()
.withName(testObjectName2)
.withType(TestObjectPropertyType.FILE_TYPE)
.withStrategy(TestObjectPropertyStrategy.OPTIONAL);
TestObjectProperty property2 = builder2.build();
testObject2.addProperty(property2);
verifyFiles(testObject1, testObject2, message);
}
}
```
使用上述代码,可以比较两个文件的内容是否相同。
阅读全文
相关推荐
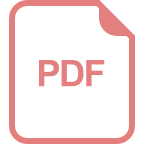
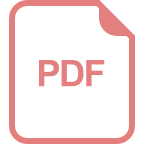



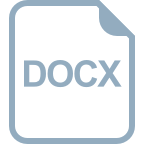
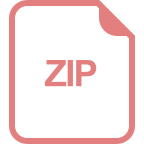
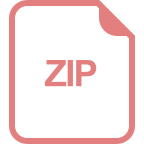
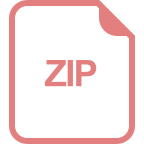
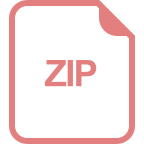
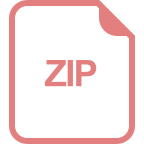
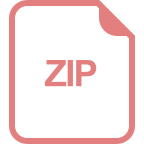
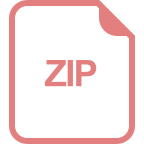