movable-view 缩放 如何实现
时间: 2023-07-20 15:24:57 浏览: 103
要在 `movable-view` 组件中实现缩放功能,可以通过以下步骤实现:
1. 在 `movable-view` 中嵌套一个 `view` 组件,并设置 `view` 的初始样式和缩放比例。
2. 在 `movable-view` 的 `touchstart` 事件中记录当前手指的位置和 `view` 的初始尺寸和位置。
3. 在 `movable-view` 的 `touchmove` 事件中计算手指移动的距离和缩放比例,根据移动距离和缩放比例计算 `view` 的样式,并设置 `view` 的样式。
4. 在 `movable-view` 的 `touchend` 事件中重置 `view` 的尺寸和位置。
下面是一个实现 `movable-view` 缩放功能的示例代码:
```html
<!-- wxml -->
<view class="container">
<movable-view class="movable-view" direction="all" bindtouchstart="onTouchStart" bindtouchmove="onTouchMove" bindtouchend="onTouchEnd">
<view class="content" style="{{viewStyle}}"></view>
</movable-view>
</view>
```
```javascript
// js
Page({
data: {
startTouches: [],
viewStyle: 'width: 200px; height: 200px; transform: scale(1);',
viewWidth: 200,
viewHeight: 200,
viewLeft: 0,
viewTop: 0,
},
onTouchStart(e) {
const { pageX, pageY } = e.touches[0]
const { viewWidth, viewHeight } = this.data
this.setData({
startTouches: [{ x: pageX, y: pageY }],
viewStyle: `width: ${viewWidth}px; height: ${viewHeight}px; transform: scale(1);`,
})
},
onTouchMove(e) {
const { pageX, pageY } = e.touches[0]
const { startTouches, viewWidth, viewHeight, viewLeft, viewTop } = this.data
if (e.touches.length === 2) {
const startDistance = Math.sqrt(Math.pow(startTouches[1].x - startTouches[0].x, 2) + Math.pow(startTouches[1].y - startTouches[0].y, 2))
const endDistance = Math.sqrt(Math.pow(e.touches[1].pageX - e.touches[0].pageX, 2) + Math.pow(e.touches[1].pageY - e.touches[0].pageY, 2))
const scale = endDistance / startDistance
const width = viewWidth * scale
const height = viewHeight * scale
const left = viewLeft - (width - viewWidth) / 2
const top = viewTop - (height - viewHeight) / 2
this.setData({
viewStyle: `width: ${width}px; height: ${height}px; transform: scale(${scale}); left: ${left}px; top: ${top}px;`,
})
} else {
const deltaX = pageX - startTouches[0].x
const deltaY = pageY - startTouches[0].y
const left = viewLeft + deltaX
const top = viewTop + deltaY
this.setData({
viewStyle: `width: ${viewWidth}px; height: ${viewHeight}px; transform: scale(1); left: ${left}px; top: ${top}px;`,
})
}
},
onTouchEnd(e) {
const { viewWidth, viewHeight, viewLeft, viewTop } = this.data
this.setData({
startTouches: [],
viewStyle: `width: ${viewWidth}px; height: ${viewHeight}px; transform: scale(1); left: ${viewLeft}px; top: ${viewTop}px;`,
})
},
})
```
在上面的代码中,我们在 `movable-view` 中嵌套了一个 `view` 组件,并将其样式设置为可缩放的。在 `touchstart` 事件中记录了当前手指的位置和 `view` 的初始尺寸和位置。在 `touchmove` 事件中,如果手指数量为 2,就计算出手指之间的距离和缩放比例,并根据缩放比例计算出 `view` 的样式。如果手指数量为 1,就计算出手指移动的距离,并根据移动距离计算出 `view` 的样式。在 `touchend` 事件中,重置 `view` 的尺寸和位置。
相关推荐
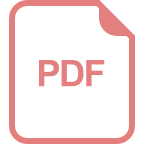
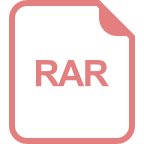
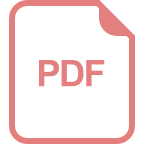














