用python写一个带gui界面的可以自定义词云形状的词云生成器并对它的代码进行解释
时间: 2024-04-30 17:17:44 浏览: 96
好的,我来为您解答。首先,我们需要安装 `wordcloud` 和 `tkinter` 这两个 Python 库,可以使用以下命令进行安装:
```python
!pip install wordcloud
```
`tkinter` 库是 Python 自带的 GUI 库,无需安装。
接下来,我们需要编写代码:
```python
import tkinter as tk
import tkinter.filedialog
from PIL import Image, ImageTk
from wordcloud import WordCloud, STOPWORDS
class WordCloudGenerator:
def __init__(self, root):
self.root = root
self.root.title("词云生成器")
self.root.geometry("600x600")
self.file_path = ""
self.shape_path = ""
# 设置输入框
input_label = tk.Label(self.root, text="请输入要生成词云的文本:")
input_label.pack(pady=10)
self.input_text = tk.Text(self.root, height=10)
self.input_text.pack()
# 设置选择文件按钮
file_button = tk.Button(self.root, text="选择文件", command=self.select_file)
file_button.pack(pady=10)
# 设置选择形状按钮
shape_button = tk.Button(self.root, text="选择形状", command=self.select_shape)
shape_button.pack(pady=10)
# 设置生成词云按钮
generate_button = tk.Button(self.root, text="生成词云", command=self.generate_wordcloud)
generate_button.pack(pady=10)
# 设置显示图片的 Label
self.image_label = tk.Label(self.root)
self.image_label.pack()
def select_file(self):
self.file_path = tk.filedialog.askopenfilename()
def select_shape(self):
self.shape_path = tk.filedialog.askopenfilename()
def generate_wordcloud(self):
text = self.input_text.get("1.0", "end-1c")
stopwords = set(STOPWORDS)
if self.file_path:
with open(self.file_path, "r") as f:
text += f.read()
if self.shape_path:
mask = Image.open(self.shape_path)
mask = mask.convert("L")
mask = mask.resize((600, 600))
wc = WordCloud(background_color="white", stopwords=stopwords, mask=mask)
else:
wc = WordCloud(background_color="white", stopwords=stopwords)
wc.generate(text)
image = wc.to_image()
photo = ImageTk.PhotoImage(image)
self.image_label.configure(image=photo)
self.image_label.image = photo
if __name__ == "__main__":
root = tk.Tk()
app = WordCloudGenerator(root)
root.mainloop()
```
以上代码实现了一个简单的 GUI 界面,包含以下功能:
1. 输入要生成词云的文本;
2. 选择文件,将文件中的文本内容追加到输入框中;
3. 选择形状,生成的词云将以该形状为背景;
4. 点击生成词云按钮,生成词云并显示在界面上。
代码解释:
1. `WordCloudGenerator` 类是我们的主界面,使用了 `tkinter` 库创建了一个窗口;
2. 在 `__init__` 方法中,我们设置了界面的标题、大小等属性,并创建了输入框、选择文件按钮、选择形状按钮、生成词云按钮和显示图片的 Label;
3. `select_file` 方法用于选择文件,将选择的文件中的文本内容追加到输入框中;
4. `select_shape` 方法用于选择形状,将选择的形状图片作为词云的背景;
5. `generate_wordcloud` 方法用于生成词云,首先获取输入框中的文本内容,然后将文件中的文本内容追加到其中,接着根据选择的形状创建词云对象,最后将词云对象生成的图片显示在界面上。
希望这能帮助到您,有任何问题欢迎继续咨询。
阅读全文
相关推荐
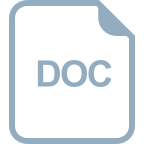
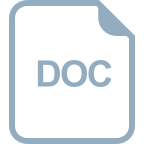
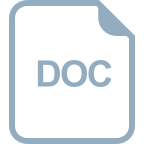
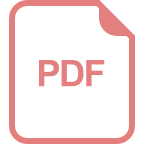
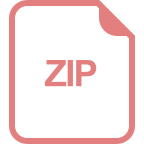
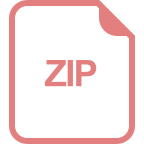
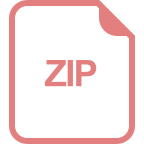
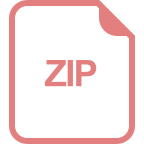
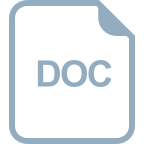
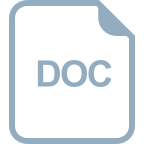
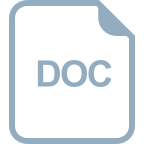







