python 通过win32自动发送邮件,将同一封邮件分成多次发给多位收件人
时间: 2024-03-03 08:48:12 浏览: 67
可以使用Python中的smtplib和email库来实现通过win32自动发送邮件的功能,并且可以将同一封邮件分成多次发送给多个收件人。以下是一段示例代码:
```python
import win32com.client as win32
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.image import MIMEImage
# 邮件相关信息
smtp_server = 'smtp.example.com'
smtp_port = 25
sender = 'sender@example.com'
password = 'password'
# 收件人列表
recipients = ['recipient1@example.com', 'recipient2@example.com', 'recipient3@example.com']
# 邮件内容
msg = MIMEMultipart()
msg['Subject'] = '邮件主题'
msg['From'] = sender
msg['To'] = ', '.join(recipients)
text = MIMEText('邮件正文')
msg.attach(text)
# 发送邮件
server = smtplib.SMTP(smtp_server, smtp_port)
server.login(sender, password)
# 将邮件分成多份发送
for recipient in recipients:
msg['To'] = recipient
server.sendmail(sender, recipient, msg.as_string())
server.quit()
```
在以上示例代码中,我们首先定义了邮件相关的信息,包括SMTP服务器、SMTP端口、发件人邮箱和密码。然后定义了收件人列表和邮件内容,包括邮件主题和正文。最后通过for循环将同一封邮件分别发送给每个收件人。在每次循环中,我们需要更新邮件头中的收件人信息,并使用`server.sendmail()`方法来发送邮件。最后,我们使用`server.quit()`方法关闭SMTP连接。
阅读全文
相关推荐
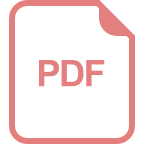
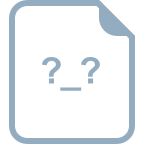
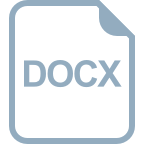
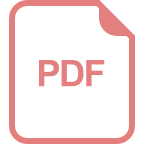
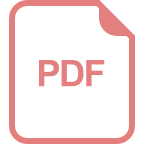
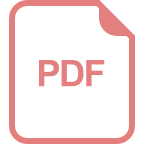
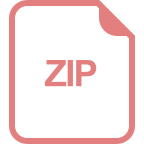
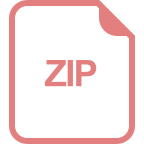
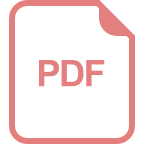
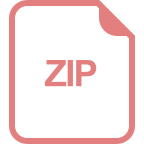
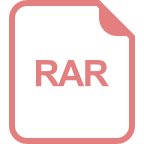
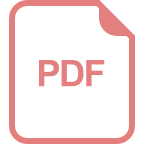
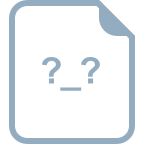
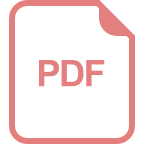