<template #="{ row, $index }"> <el-button type="primary" size="small" icon="User" @click="setRole(row)">分配角色</el-button> <el-button type="primary" size="small" icon="Edit" @click="updateUser(row)">编辑</el-button> <!-- el-popconfirm 组件是一个弹出确认框,当用户点击“删除”按钮时,会弹出一个确认框来提示用户是否确定要删除该用户 --> <el-popconfirm :title="`你确定要删除${row.username}?`" width="260px" @confirm="deleteUser(row.id)"> <template #reference> <el-button type="primary" size="small" icon="Delete">删除</el-button> </template> </el-popconfirm> </template> 上述代码使用不是插槽有什么实际区别
时间: 2024-03-30 07:38:56 浏览: 147
这段代码使用了 Vue.js 的 template syntax 中的解构赋值语法 (destructuring assignment syntax)。通过这种方式,我们可以将 template 中的 row 和 $index 两个对象解构并传递给当前组件。在这个例子中,row 表示当前循环的数据项,$index 表示当前数据项的索引。
而使用插槽,我们需要在父组件中定义插槽,并且在子组件中使用插槽名称来引用它。在这个例子中,我们可以将这段代码封装成一个组件,并在父组件中使用插槽来引用这个组件,实现相同的效果。
使用解构赋值语法的好处是代码更加简洁,同时也方便我们在 template 中使用当前数据项的属性。而使用插槽的好处在于我们可以更好地封装组件,并在父组件中复用它。
相关问题
<template #="{ row, $index }"> <el-button type="primary" size="small" icon="User" @click="setRole(row)">分配角色</el-button> <el-button type="primary" size="small" icon="Edit" @click="updateUser(row)">编辑</el-button> <el-popconfirm :title="`你确定要删除${row.username}?`" width="260px" @confirm="deleteUser(row.id)"> <template #reference> <el-button type="primary" size="small" icon="Delete">删除</el-button> </template> </el-popconfirm> </template>详细解释上述代码
这段代码是一个 Vue.js 组件中使用 Element UI 组件库进行渲染的模板代码。它使用了 Vue.js 3 中的解构插槽语法,并结合了 Element UI 组件的特性来实现一个用户列表的操作界面。
具体来说,这个模板中包含了三个操作按钮,分别是“分配角色”、“编辑”和“删除”。这些按钮的点击事件都会触发组件中对应的方法,以完成相应的操作。
这个模板的核心在于 `template` 标签中的 `#="{ row, $index }"` 属性。这个属性使用了解构语法,将插槽内容解构为一个对象并命名为 `row` 和 `$index`。这个对象中包含了当前循环的数据和索引,可以在插槽内容中使用。
在插槽内容中,使用了 Element UI 中的 `el-button` 和 `el-popconfirm` 组件来渲染操作按钮。其中,`el-popconfirm` 组件是一个弹出确认框,当用户点击“删除”按钮时,会弹出一个确认框来提示用户是否确定要删除该用户。
总的来说,这段代码实现了一个用户列表的操作界面,让用户可以方便地对列表中的每个用户进行编辑、删除和角色分配等操作。
将以下vue+element ui代码转换成纯html+element ui代码:<template> <el-tabs v-model="activeName" class="demo-tabs" @tab-click="handleClick"> <el-tab-pane label="User" name="first"> <el-table :data="filterTableData" style="width: 100%"> <el-table-column label="Date" prop="date" /> <el-table-column label="Name" prop="name" /> <el-table-column align="right"> <template #header> <el-input v-model="search" size="small" placeholder="Type to search" /> </template> <template #default="scope"> <el-button size="small" @click="handleEdit(scope.$index, scope.row)" >Edit</el-button > <el-button size="small" type="danger" @click="handleDelete(scope.$index, scope.row)" >Delete</el-button > </template> </el-table-column> </el-table> </el-tab-pane> <el-tab-pane label="Config" name="second"> <el-table :data="filterTableData" style="width: 100%"> <el-table-column label="Date" prop="date" /> <el-table-column label="Name" prop="name" /> <el-table-column align="right"> <template #header> <el-input v-model="search" size="small" placeholder="Type to search" /> </template> <template #default="scope"> <el-button size="small" @click="handleEdit(scope.$index, scope.row)" >Edit</el-button > <el-button size="small" type="danger" @click="handleDelete(scope.$index, scope.row)" >Delete</el-button > </template> </el-table-column> </el-table></el-tab-pane> <el-tab-pane label="Role" name="third"> <el-table :data="filterTableData" style="width: 100%"> <el-table-column label="Date" prop="date" /> <el-table-column label="Name" prop="name" /> <el-table-column align="right"> <template #header> <el-input v-model="search" size="small" placeholder="Type to search" /> </template> <template #default="scope"> <el-button size="small" @click="handleEdit(scope.$index, scope.row)" >Edit</el-button > <el-button size="small" type="danger" @click="handleDelete(scope.$index, scope.row)" >Delete</el-button > </template> </el-table-column> </el-table></el-tab-pane> <el-tab-pane label="Task" name="fourth"> <el-table :data="filterTableData" style="width: 100%"> <el-table-column label="Date" prop="date" /> <el-table-column label="Name" prop="name" /> <el-table-column align="right"> <template #header> <el-input v-model="search" size="small" placeholder="Type to search" /> </template> <template #default="scope"> <el-button size="small" @click="handleEdit(scope.$index, scope.row)" >Edit</el-button > <el-button size="small" type="danger" @click="handleDelete(scope.$index, scope.row)" >Delete</el-button > </template> </el-table-column> </el-table></el-tab-pane> </el-tabs> </template> <script lang="ts" setup> import { computed, ref } from 'vue' interface User { date: string name: string address: string } const search = ref('') const filterTableData = computed(() => tableData.filter( (data) => !search.value || data.name.toLowerCase().includes(search.value.toLowerCase()) ) ) const handleEdit = (index: number, row: User) => { console.log(index, row) } const handleDelete = (index: number, row: User) => { console.log(index, row) } const tableData: User[] = [ { date: '2016-05-03', name: 'Tom', address: 'No. 189, Grove St, Los Angeles', }, { date: '2016-05-02', name: 'John', address: 'No. 189, Grove St, Los Angeles', }, { date: '2016-05-04', name: 'Morgan', address: 'No. 189, Grove St, Los Angeles', }, { date: '2016-05-01', name: 'Jessy', address: 'No. 189, Grove St, Los Angeles', }, ] </script> <style> .demo-tabs > .el-tabs__content { padding: 32px; color: #6b778c; font-size: 32px; font-weight: 600; } </style>
以下是转换后的纯HTML+Element UI代码:
```html
<div class="demo-tabs el-tabs">
<div class="el-tabs__header">
<div class="el-tabs__nav-wrap">
<div role="tab" aria-controls="pane-first" :aria-selected="activeName==='first' ? 'true' : 'false'" class="el-tabs__item" :class="{ 'is-active': activeName==='first' }" @click="activeName='first'">User</div>
<div role="tab" aria-controls="pane-second" :aria-selected="activeName==='second' ? 'true' : 'false'" class="el-tabs__item" :class="{ 'is-active': activeName==='second' }" @click="activeName='second'">Config</div>
<div role="tab" aria-controls="pane-third" :aria-selected="activeName==='third' ? 'true' : 'false'" class="el-tabs__item" :class="{ 'is-active': activeName==='third' }" @click="activeName='third'">Role</div>
<div role="tab" aria-controls="pane-fourth" :aria-selected="activeName==='fourth' ? 'true' : 'false'" class="el-tabs__item" :class="{ 'is-active': activeName==='fourth' }" @click="activeName='fourth'">Task</div>
</div>
</div>
<div class="el-tabs__content">
<div role="tabpanel" aria-hidden="false" id="pane-first" class="el-tab-pane" :class="{ 'is-active': activeName==='first' }">
<table class="el-table" style="width: 100%">
<thead class="el-table__header">
<tr>
<th class="el-table_1_column_1">Date</th>
<th class="el-table_1_column_2">Name</th>
<th class="el-table_1_column_3">Operation</th>
</tr>
</thead>
<tbody class="el-table__body">
<tr v-for="(item, index) in filterTableData" :key="index">
<td>{{ item.date }}</td>
<td>{{ item.name }}</td>
<td class="el-table_1_column_3">
<button class="el-button el-button--small" @click="handleEdit(index, item)">Edit</button>
<button class="el-button el-button--small el-button--danger" @click="handleDelete(index, item)">Delete</button>
</td>
</tr>
</tbody>
</table>
</div>
<div role="tabpanel" aria-hidden="true" id="pane-second" class="el-tab-pane" :class="{ 'is-active': activeName==='second' }">
<table class="el-table" style="width: 100%">
<thead class="el-table__header">
<tr>
<th class="el-table_2_column_1">Date</th>
<th class="el-table_2_column_2">Name</th>
<th class="el-table_2_column_3">Operation</th>
</tr>
</thead>
<tbody class="el-table__body">
<tr v-for="(item, index) in filterTableData" :key="index">
<td>{{ item.date }}</td>
<td>{{ item.name }}</td>
<td class="el-table_2_column_3">
<button class="el-button el-button--small" @click="handleEdit(index, item)">Edit</button>
<button class="el-button el-button--small el-button--danger" @click="handleDelete(index, item)">Delete</button>
</td>
</tr>
</tbody>
</table>
</div>
<div role="tabpanel" aria-hidden="true" id="pane-third" class="el-tab-pane" :class="{ 'is-active': activeName==='third' }">
<table class="el-table" style="width: 100%">
<thead class="el-table__header">
<tr>
<th class="el-table_3_column_1">Date</th>
<th class="el-table_3_column_2">Name</th>
<th class="el-table_3_column_3">Operation</th>
</tr>
</thead>
<tbody class="el-table__body">
<tr v-for="(item, index) in filterTableData" :key="index">
<td>{{ item.date }}</td>
<td>{{ item.name }}</td>
<td class="el-table_3_column_3">
<button class="el-button el-button--small" @click="handleEdit(index, item)">Edit</button>
<button class="el-button el-button--small el-button--danger" @click="handleDelete(index, item)">Delete</button>
</td>
</tr>
</tbody>
</table>
</div>
<div role="tabpanel" aria-hidden="true" id="pane-fourth" class="el-tab-pane" :class="{ 'is-active': activeName==='fourth' }">
<table class="el-table" style="width: 100%">
<thead class="el-table__header">
<tr>
<th class="el-table_4_column_1">Date</th>
<th class="el-table_4_column_2">Name</th>
<th class="el-table_4_column_3">Operation</th>
</tr>
</thead>
<tbody class="el-table__body">
<tr v-for="(item, index) in filterTableData" :key="index">
<td>{{ item.date }}</td>
<td>{{ item.name }}</td>
<td class="el-table_4_column_3">
<button class="el-button el-button--small" @click="handleEdit(index, item)">Edit</button>
<button class="el-button el-button--small el-button--danger" @click="handleDelete(index, item)">Delete</button>
</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
```
需要注意的是,Element UI的CSS样式需要在HTML文件中引入。此外,上述代码中的`activeName`、`search`、`filterTableData`、`handleEdit`和`handleDelete`需要在JavaScript中定义和实现。
阅读全文
相关推荐
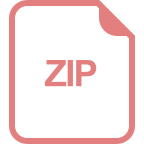
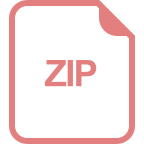
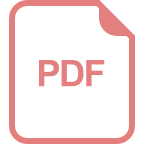



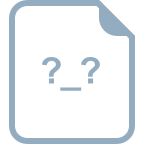
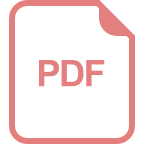
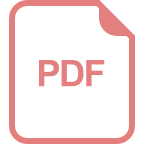
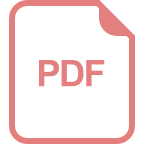
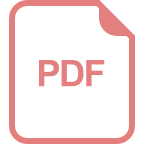
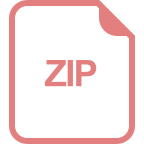
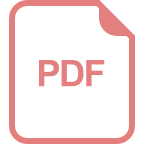
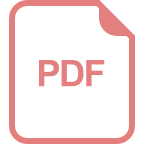
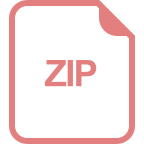
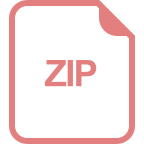
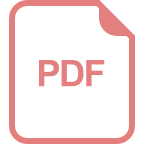