Biopython常用命令
时间: 2023-08-25 10:07:43 浏览: 53
1.读取序列文件:
```python
from Bio import SeqIO
record = SeqIO.read("example.fasta", "fasta")
print(record.id, "\n", record.seq)
```
2.写序列文件:
```python
from Bio.Seq import Seq
from Bio.SeqRecord import SeqRecord
from Bio import SeqIO
seq = Seq("ATCG")
record = SeqRecord(seq, id="example", description="example sequence")
SeqIO.write(record, "example.fasta", "fasta")
```
3.翻译DNA序列:
```python
from Bio.Seq import Seq
from Bio.Alphabet import generic_dna
dna_seq = Seq("ATGGCCATTGTAATGGGCCGCTGAAAGGGTGCCCGATAG", generic_dna)
protein_seq = dna_seq.translate()
print(protein_seq)
```
4.比对序列:
```python
from Bio.Seq import Seq
from Bio import pairwise2
seq1 = Seq("ATCGATCG")
seq2 = Seq("ATCGATAG")
alignments = pairwise2.align.globalxx(seq1, seq2)
for alignment in alignments:
print(alignment)
```
5.计算DNA序列碱基组成:
```python
from Bio.Seq import Seq
from Bio.SeqUtils import GC, nt_search
seq = Seq("ATCGATCG")
gc_content = GC(seq)
print(gc_content)
```
6.计算蛋白质序列氨基酸组成:
```python
from Bio.Seq import Seq
from Bio.SeqUtils import ProtParam
protein_seq = Seq("MKQHKAMIVALIVICITAVVAALVTRKDLCEVHIRTGQTEVAVF")
protein_param = ProtParam.ProteinAnalysis(str(protein_seq))
print(protein_param.count_amino_acids())
```
7.解析BLAST结果:
```python
from Bio.Blast import NCBIXML
result_handle = open("blast_output.xml")
blast_records = NCBIXML.parse(result_handle)
for blast_record in blast_records:
for alignment in blast_record.alignments:
for hsp in alignment.hsps:
print("Query:", blast_record.query)
print("Subject:", alignment.title)
print("Length:", alignment.length)
print("E-value:", hsp.expect)
print("Score:", hsp.score)
print("Query sequence:", hsp.query)
print("Subject sequence:", hsp.sbjct)
```
8.计算序列间距离:
```python
from Bio.Align.Applications import ClustalwCommandline
from Bio import AlignIO
from Bio.Phylo.TreeConstruction import DistanceCalculator
from Bio import Phylo
input_file = "example.fasta"
output_file = "example.aln"
clustalw_cmd = ClustalwCommandline("clustalw2", infile=input_file, outfile=output_file)
clustalw_cmd()
alignment = AlignIO.read(output_file, "clustal")
calculator = DistanceCalculator("identity")
dm = calculator.get_distance(alignment)
print(dm)
```
9.构建进化树:
```python
from Bio.Align.Applications import ClustalwCommandline
from Bio import AlignIO
from Bio.Phylo.TreeConstruction import DistanceCalculator, DistanceTreeConstructor
from Bio import Phylo
input_file = "example.fasta"
output_file = "example.aln"
clustalw_cmd = ClustalwCommandline("clustalw2", infile=input_file, outfile=output_file)
clustalw_cmd()
alignment = AlignIO.read(output_file, "clustal")
calculator = DistanceCalculator("identity")
dm = calculator.get_distance(alignment)
constructor = DistanceTreeConstructor()
tree = constructor.build_tree(dm)
Phylo.draw_ascii(tree)
```
相关推荐









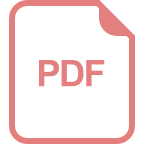