base_dir = path(__file__).resolve().parent.parent
时间: 2023-05-01 13:03:40 浏览: 152
这行代码表示,将当前文件(__file__)所在的路径(path)获取出来,并通过resolve()方法获取绝对路径,然后再通过parent.parent获取到该路径的上上一级目录,即为base_dir。
相关问题
def __init__( self, volume_path: Union[str, Path], minimum_file_number: int = 64, extensions: Tuple = ( ".cb", ".png", ".tif", ".tiff", ".jpg", ".jpeg", ), volume_info_file_name: str = VOLUME_INFO_FILE_NAME, ) -> None: self.volume_path = Path(volume_path).resolve() self.minimum_file_number = minimum_file_number self.extensions = extensions self.volume_info_path = Path(self.volume_path, volume_info_file_name) self.__image_files: List[Path] = None assert self.volume_path.is_dir()请完整详细解释每一行代码的意思
这段代码是一个Python类的构造函数,用于初始化类的属性。以下是每一行代码的意思:
- def __init__(self, volume_path: Union[str, Path], minimum_file_number: int = 64, extensions: Tuple = (".cb", ".png", ".tif", ".tiff", ".jpg", ".jpeg", ), volume_info_file_name: str = VOLUME_INFO_FILE_NAME, ) -> None:
这是构造函数的头部,其中包括参数列表和返回值。它接收一个必要参数volume_path和三个可选参数minimum_file_number、extensions、volume_info_file_name,返回None。
- self.volume_path = Path(volume_path).resolve()
这一行代码将传入的volume_path参数转换为Path对象,并使用.resolve()方法获取绝对路径,并将其赋值给类的volume_path属性。
- self.minimum_file_number = minimum_file_number
这一行代码将minimum_file_number参数的值直接赋给类的minimum_file_number属性。
- self.extensions = extensions
这一行代码将extensions参数的值直接赋给类的extensions属性。
- self.volume_info_path = Path(self.volume_path, volume_info_file_name)
这一行代码使用已解析的volume_path和volume_info_file_name参数创建一个新的Path对象,并将其赋值给类的volume_info_path属性。
- self.__image_files: List[Path] = None
这一行代码定义了一个私有属性__image_files,使用Python类型提示说明其类型为List[Path],初始值为None。
- assert self.volume_path.is_dir()
这一行代码使用断言检查volume_path是否为一个目录,如果不是,则会抛出AssertionError。
if name == "main": parser = argparse.ArgumentParser(description="Intensity Normalizer") parser.add_argument("-s", "--src", type=str, help="source directory.") parser.add_argument("-d", "--dst", type=str, help="destination directory.") parser.add_argument( "--mm_resolution", type=float, default=0.0, help="spatial resolution [mm].", ) parser.add_argument( "--depth", type=int, default=-1, help="depth of the maximum level to be explored. Defaults to unlimited.", ) args = parser.parse_args() if args.src is None: parser.print_help() exit(0) root_src_dir: Path = Path(args.src).resolve() if not root_src_dir.is_dir(): logger.error("Indicate valid virectory path.") exit() root_dst_dir = Path( args.dst or str(root_src_dir) + "_intensity_normalized" ) mm_resolution = float(args.mm_resolution) depth = int(args.depth) volume_loading_queue = Queue() volume_loading_process = Process( target=volume_loading_func, args=(root_src_dir, root_dst_dir, depth, volume_loading_queue, logger), ) volume_loading_process.start() volume_saving_queue = Queue() volume_saving_process = Process( target=volume_saving_func, args=(volume_saving_queue, logger), ) volume_saving_process.start() while True: ( volume_path, np_volume, volume_info, ) = volume_loading_queue.get() if volume_path is None: break relative_path = volume_path.relative_to(root_src_dir) np_volume = normalize_intensity(np_volume, relative_path, logger) if mm_resolution != 0: volume_info.update({"mm_resolution": mm_resolution}) while volume_saving_queue.qsize() == 1: pass dst_path = Path( root_dst_dir, re.sub(r"cb\d{3}$", "", str(relative_path)) ) volume_saving_queue.put( (dst_path, root_dst_dir, np_volume, volume_info) ) volume_saving_queue.put((None, None, None, None))请完整详细的解释每一行的代码意思
这段代码是一个 Python 程序,主要用于进行图像的强度归一化操作。下面是每一行代码的详细解释。
```python
if name == "main":
```
这是 Python 程序的入口,判断是否为主程序。
```python
parser = argparse.ArgumentParser(description="Intensity Normalizer")
parser.add_argument("-s", "--src", type=str, help="source directory.")
parser.add_argument("-d", "--dst", type=str, help="destination directory.")
parser.add_argument("--mm_resolution", type=float, default=0.0, help="spatial resolution [mm].")
parser.add_argument("--depth", type=int, default=-1, help="depth of the maximum level to be explored. Defaults to unlimited.")
args = parser.parse_args()
```
这段代码定义了命令行选项,用于接收程序运行时传入的参数。其中 `-s` 选项指定输入文件夹路径,`-d` 选项指定输出文件夹路径,`--mm_resolution` 选项指定空间分辨率,`--depth` 选项指定文件夹遍历的深度。然后使用 `parse_args()` 方法解析参数并将结果存放在 `args` 对象中。
```python
if args.src is None:
parser.print_help()
exit(0)
```
判断是否输入了输入文件夹路径,如果没有则打印帮助信息并退出程序。
```python
root_src_dir: Path = Path(args.src).resolve()
if not root_src_dir.is_dir():
logger.error("Indicate valid virectory path.")
exit()
```
将输入文件夹路径转换成 `Path` 对象,并使用 `resolve()` 方法去除路径中的符号链接,然后判断路径是否存在且是一个文件夹,如果不是则输出错误信息并退出程序。
```python
root_dst_dir = Path(args.dst or str(root_src_dir) + "_intensity_normalized")
mm_resolution = float(args.mm_resolution)
depth = int(args.depth)
```
如果指定了输出文件夹路径,则将其转换成 `Path` 对象,否则根据输入文件夹路径生成一个默认输出文件夹路径。然后将空间分辨率和文件夹遍历深度转换成对应的数据类型。
```python
volume_loading_queue = Queue()
volume_loading_process = Process(target=volume_loading_func, args=(root_src_dir, root_dst_dir, depth, volume_loading_queue, logger))
volume_loading_process.start()
```
使用 `multiprocessing` 模块创建一个进程,其中 `volume_loading_func` 是一个函数,主要用于加载图像数据并放入消息队列中。这里将输入文件夹路径、输出文件夹路径、文件夹遍历深度、消息队列和日志记录器作为参数传入进程。
```python
volume_saving_queue = Queue()
volume_saving_process = Process(target=volume_saving_func, args=(volume_saving_queue, logger))
volume_saving_process.start()
```
同样使用 `multiprocessing` 模块创建另一个进程,其中 `volume_saving_func` 是一个函数,主要用于将归一化后的图像数据保存到输出文件夹中。这里只需将消息队列和日志记录器作为参数传入进程即可。
```python
while True:
(volume_path, np_volume, volume_info,) = volume_loading_queue.get()
if volume_path is None:
break
relative_path = volume_path.relative_to(root_src_dir)
np_volume = normalize_intensity(np_volume, relative_path, logger)
if mm_resolution != 0:
volume_info.update({"mm_resolution": mm_resolution})
while volume_saving_queue.qsize() == 1:
pass
dst_path = Path(root_dst_dir, re.sub(r"cb\d{3}$", "", str(relative_path)))
volume_saving_queue.put((dst_path, root_dst_dir, np_volume, volume_info))
volume_saving_queue.put((None, None, None, None))
```
进入无限循环,从消息队列中获取从 `volume_loading_func` 函数中发来的消息。如果消息内容中的 `volume_path` 为 `None`,则表示所有图像数据已经处理完毕,退出循环。否则,从 `volume_path` 中提取相对输入文件夹路径并调用 `normalize_intensity` 函数对图像数据进行归一化处理,并更新 `volume_info` 中的空间分辨率。然后等待消息队列中只有一个等待处理的消息,将处理后的图像数据和相应的数据信息发送到 `volume_saving_func` 函数中进行保存。最后向消息队列中发送一个空消息以表示图像数据处理完毕。
整个程序实现了对文件夹中的图像进行强度归一化操作,并支持指定空间分辨率和文件夹遍历深度等功能。
相关推荐
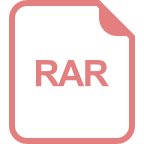













