用java完成以下需求 创建一个表示学生的类Student创建五个对象,将这5个对象序列化,并把序列化后的字节信息输出到D盘名为”序列化.txt”文件中;再将”序列化.txt”文件中对象的字节信息进行反序列化,输出到控制台中。
时间: 2024-03-20 12:40:02 浏览: 21
好的,这是一个比较基础的Java编程题,我来给你讲解一下。
首先,我们需要定义一个表示学生的类Student,可以包含以下属性:
```java
public class Student implements Serializable {
private String name;
private int age;
private String gender;
private String id;
// 构造函数、getter和setter方法
}
```
接着,我们需要创建5个学生对象,并将它们序列化成字节流,将字节流输出到文件中。代码如下:
```java
import java.io.FileOutputStream;
import java.io.ObjectOutputStream;
public class SerializeTest {
public static void main(String[] args) {
try {
Student[] students = new Student[5];
students[0] = new Student("Tom", 18, "male", "001");
students[1] = new Student("Jerry", 19, "male", "002");
students[2] = new Student("Alice", 20, "female", "003");
students[3] = new Student("Bob", 21, "male", "004");
students[4] = new Student("Cindy", 22, "female", "005");
FileOutputStream fos = new FileOutputStream("D:\\序列化.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
for (Student student : students) {
oos.writeObject(student);
}
oos.close();
fos.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
最后,我们需要将序列化后的字节流从文件中读取出来,并反序列化成学生对象,输出到控制台中。代码如下:
```java
import java.io.FileInputStream;
import java.io.ObjectInputStream;
public class DeserializeTest {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("D:\\序列化.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
for (int i = 0; i < 5; i++) {
Student student = (Student) ois.readObject();
System.out.println(student.getName() + " " + student.getAge() + " " + student.getGender() + " " + student.getId());
}
ois.close();
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上就是完成这个需求的代码实现。需要注意的是,要让Student类实现Serializable接口,才能进行序列化和反序列化操作。
相关推荐
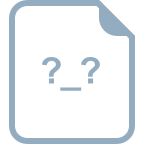
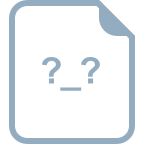














