C++获取文件的最后修改时间戳
时间: 2024-03-12 20:47:37 浏览: 172
在C++中,你可以使用`stat`函数来获取文件的最后修改时间戳。下面是一个示例代码:
```c++
#include <iostream>
#include <sys/stat.h>
#include <ctime>
int main() {
const char* filename = "path/to/your/file";
struct stat result;
if (stat(filename, &result) == 0) {
std::time_t last_modified = result.st_mtime;
std::cout << "Last modified time: " << last_modified << std::endl;
} else {
std::cout << "Failed to get file info" << std::endl;
}
return 0;
}
```
其中`filename`是你要获取最后修改时间的文件路径。`stat`函数将文件信息保存在`struct stat`结构体中,你可以从中获取最后修改时间戳。最后修改时间戳是一个`time_t`类型的整数,你可以使用`std::ctime`函数将其转换为可读的时间字符串。
相关问题
C++怎么获取文件或文件夹的最后修改时间并转换年、月、日、时、分的形式存储
在C++中,你可以使用`<filesystem>`库(C++17及以上版本)来获取文件或文件夹的最后修改时间,并通过`std::chrono`库将其转换为特定格式。以下是一个简单的示例:
```cpp
#include <iostream>
#include <filesystem>
#include <chrono>
// 时间戳到字符串函数
std::string format_time(const std::time_t& time) {
auto now = std::chrono::system_clock::to_time_t(std::chrono::system_clock::now());
char buffer[80];
std::strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M", std::localtime(&time));
return buffer;
}
int main() {
try {
// 获取当前目录
std::filesystem::path dir_path(".");
for (const auto& entry : std::filesystem::directory_iterator(dir_path)) {
if (entry.is_regular_file()) {
// 文件存在,获取最后修改时间
std::time_t last_modification_time = entry.last_write_time().count();
std::string formatted_time = format_time(last_modification_time);
std::cout << "File: " << entry.path() << ", Last Modified: " << formatted_time << std::endl;
}
}
} catch (const std::filesystem::filesystem_error& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
这个程序会遍历指定目录下的所有文件,获取每个文件的最后修改时间(以`std::time_t`表示),然后使用`std::strftime`将时间戳转换成年-月-日-时-分的形式。
如何在Visual C++中编程获取并修改文件的创建时间、最后访问时间和最后写入时间?请提供详细代码示例。
在Windows平台上,使用Visual C++编程修改文件的时间戳属性是一个常见的需求。为此,你可以通过Windows API来实现这一功能。首先,你需要了解`WIN32_FIND_DATA`结构体,它能够存储文件的详细信息,包括文件的时间属性。然后,通过`FindFirstFile`和`FindNextFile`函数可以遍历文件系统并获取文件的这些信息。修改时间戳时,你需要使用`SetFileTime`函数来更新文件的创建时间、最后访问时间和最后写入时间。以下是具体的步骤和代码示例:
参考资源链接:[Visual C++ 实现文件属性修改:创建时间与访问时间](https://wenku.csdn.net/doc/17ogwyv9hc?spm=1055.2569.3001.10343)
1. 包含必要的头文件并定义文件路径:
```cpp
#include <windows.h>
#include <tchar.h>
TCHAR *filePath = _T(
参考资源链接:[Visual C++ 实现文件属性修改:创建时间与访问时间](https://wenku.csdn.net/doc/17ogwyv9hc?spm=1055.2569.3001.10343)
阅读全文
相关推荐
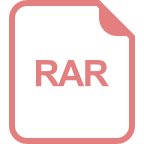
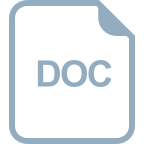
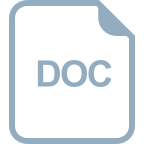
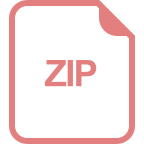
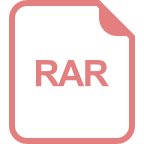
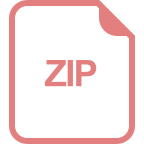
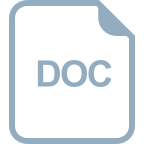
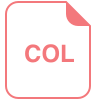
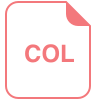
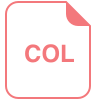
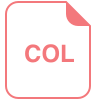
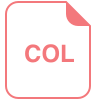

