C++调用Windows WPI打开串口
时间: 2024-09-13 18:14:02 浏览: 86
在C++中调用Windows API(WinAPI)来打开串口是一个涉及到Win32编程的任务。Windows提供了串口通信的API,主要集中在`<windows.h>`头文件中。以下是使用WinAPI打开串口的基本步骤:
1. 打开串口:使用`CreateFile`函数来打开串口设备。这个函数需要传入串口的名称(例如"COM1"),以及一系列标志来指示操作方式(如读/写等)。
2. 配置串口:串口打开之后,可能需要配置串口的属性,如波特率、数据位、停止位、校验位等。这可以通过`GetCommState`和`SetCommState`函数,与一个`DCB`(设备控制块)结构体一起使用来完成。
3. 进行串口通信:配置好串口后,就可以使用`ReadFile`和`WriteFile`等函数进行数据的读写操作了。
4. 关闭串口:完成通信后,应当使用`CloseHandle`函数关闭串口句柄,释放资源。
以下是一个简单的示例代码片段,展示如何打开和配置串口:
```cpp
#include <windows.h>
#include <iostream>
int main() {
// 打开串口
HANDLE hSerial = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, 0, 0, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, 0);
if (hSerial == INVALID_HANDLE_VALUE) {
std::cerr << "Error opening serial port" << std::endl;
return 1;
}
// 配置串口参数(示例设置波特率为9600)
DCB dcbSerialParams = {0};
dcbSerialParams.DCBlength = sizeof(dcbSerialParams);
if (!GetCommState(hSerial, &dcbSerialParams)) {
std::cerr << "Error getting serial port state" << std::endl;
CloseHandle(hSerial);
return 1;
}
dcbSerialParams.BaudRate = CBR_9600;
dcbSerialParams.ByteSize = 8;
dcbSerialParams.StopBits = ONESTOPBIT;
dcbSerialParams.Parity = NOPARITY;
if (!SetCommState(hSerial, &dcbSerialParams)) {
std::cerr << "Error setting serial port state" << std::endl;
CloseHandle(hSerial);
return 1;
}
// 串口通信操作...
// 关闭串口
CloseHandle(hSerial);
return 0;
}
```
阅读全文
相关推荐












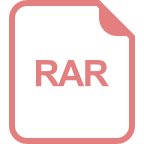




