在linux中用C语言编写程序,以只读的方式打开/home/file1,并打印file1对应的文件
时间: 2024-10-19 17:09:48 浏览: 17
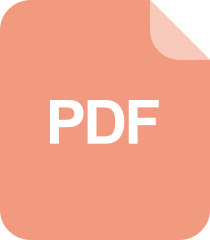
嵌入式系统/ARM技术中的 在C语言中用ASSERT调试的八个技巧
在Linux中使用C语言编写程序,以只读方式打开`/home/file1`并打印其内容,你可以参考以下步骤:
```c
#include <stdio.h>
#include <fcntl.h> // for open() system call
#include <unistd.h> // for read() and close()
#include <errno.h> // to handle errors
int main() {
int fd; // file descriptor
char buffer[4096]; // buffer to store the contents of the file
const char *filename = "/home/file1";
// 打开文件,O_RDONLY表示只读模式
if ((fd = open(filename, O_RDONLY)) == -1) { // 如果打开失败
perror("Failed to open file");
return 1;
}
// 试图读取文件内容
ssize_t bytes_read = read(fd, buffer, sizeof(buffer));
if (bytes_read == -1) { // 如果读取失败
perror("Error reading from file");
close(fd); // 确保关闭文件描述符
return 1;
} else if (bytes_read == 0) { // 文件结束(空文件)
printf("File is empty\n");
} else {
// 输出文件内容
printf("Content of %s:\n", filename);
printf("%.*s\n", (int)bytes_read, buffer);
}
// 关闭文件
close(fd);
return 0;
}
```
在这个示例中,我们首先打开文件,然后尝试从它读取数据。如果一切顺利,我们会打印出文件的内容。请注意,实际运行此程序前请确认`/home/file1`路径下有有效的文件。
阅读全文
相关推荐
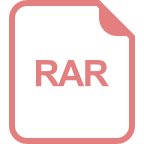
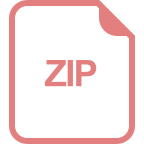

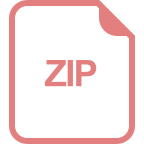
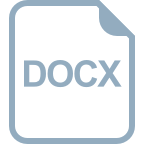
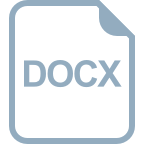
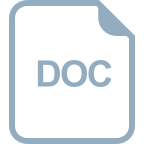
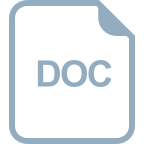
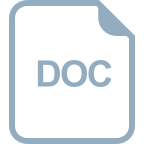
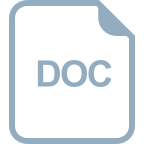
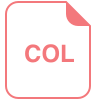




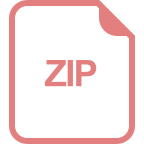
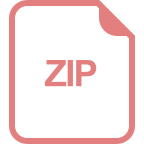