编写函数在字符串中删去字符串
时间: 2023-06-10 14:04:42 浏览: 47
中的特定字符,该函数的输入应该是原字符串和要删除的字符。
好的,您可以使用 Python 编写如下的函数来删除一个字符串中的特定字符:
```python
def remove_char(input_string: str, char_to_remove: str) -> str:
"""
Remove all occurrences of a particular character in a string.
:param input_string: The original string
:param char_to_remove: The character to be removed from the string
:return: A new string with all occurrences of the target character removed
"""
output_string = ""
for char in input_string:
if char != char_to_remove:
output_string += char
return output_string
```
您可以将原始字符串和要删除的字符作为参数传递给此函数。它会遍历字符串中的每个字符并检查它是否与要删除的字符相同。如果字符不等于此字符,则它将被添加到输出字符串中。该函数将返回一个新的字符串,其中所有出现的目标字符都已被删除。
相关问题
c语言编写函数可以删除字符串中给定字符
是的,可以使用C语言编写一个函数来删除字符串中给定的字符。以下是一个示例函数:
```c
void removeChar(char *str, char c) {
int i, j;
for (i = 0, j = 0; str[i] != '\0'; i++) {
if (str[i] != c) {
str[j++] = str[i];
}
}
str[j] = '\0';
}
```
这个函数接受两个参数:要操作的字符串和要删除的字符。它使用两个索引变量 `i` 和 `j` 来遍历字符串,将不等于要删除字符 `c` 的字符复制到 `j` 索引位置上,最后在字符串末尾加上一个空字符 `\0`。
例如,如果我们想要从字符串 `"Hello World!"` 中删除字符 `'o'`,我们可以这样调用该函数:
```c
char str[] = "Hello World!";
removeChar(str, 'o');
printf("%s\n", str); // 输出 "Hell Wrld!"
```
编写fun函数删除字符串中的数字字符
下面是一个在 Python 中删除字符串中的数字字符的函数示例:
```
def fun(s):
return ''.join([c for c in s if not c.isdigit()])
```
该函数接收一个字符串作为输入,通过使用 `isdigit()` 函数判断每个字符是否为数字字符,并在循环中删除该字符,最后使用 `join()` 函数将剩余的字符拼接成新的字符串并返回。
相关推荐
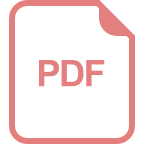
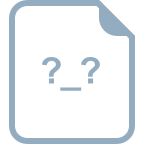
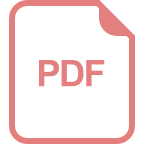












