如何历遍DataFrame数据类型
时间: 2023-07-25 15:27:15 浏览: 44
可以使用pandas库中的iterrows()方法来历遍DataFrame数据类型。这个方法可以将DataFrame中的每一行作为一个元组返回,元组的第一个元素是行号,第二个元素是该行的数据。以下是使用iterrows()方法历遍DataFrame的示例代码:
```python
import pandas as pd
# 创建一个DataFrame
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
# 使用iterrows()方法历遍DataFrame
for index, row in df.iterrows():
print(f"Row {index}: {row['name']} is {row['age']} years old.")
```
输出结果如下:
```
Row 0: Alice is 25 years old.
Row 1: Bob is 30 years old.
Row 2: Charlie is 35 years old.
```
相关问题
dataframe 数据类型转换
有三种方法可以将DataFrame数据类型转换为其他类型:
1. 使用astype()方法:可以将整个DataFrame转换为指定的数据类型,例如将所有列转换为字符串类型:dataframe = dataframe.astype(str)。也可以将某列转换为特定的数据类型,例如将某列转换为浮点型和整型:dataframe = dataframe.astype({'column1':'float','column2':'int'})
2. 使用apply函数或者map函数:可以对DataFrame的某一列进行映射操作,例如将某一列的数据转换为浮点型:data['column'] = data['column'].map(lambda x:float(x))
3. 在读取数据的时候,指定数据类型:例如在读取csv文件时,可以指定某些列的数据类型为字符串:data = pd.read_csv('file.csv', dtype={'column1':str,'column2':str})
dataframe数据类型
DataFrame 是 Pandas 库中的一个数据结构,用于处理结构化数据。它类似于表格,由行和列构成。每列可以有不同的数据类型,例如整数、浮点数、字符串等。DataFrame 便于对数据进行操作和分析。
在 Pandas 中,可以使用以下方式创建 DataFrame:
1. 从列表、字典或 NumPy 数组创建:
```python
import pandas as pd
# 从列表创建 DataFrame
data = [['Alice', 25], ['Bob', 30], ['Charlie', 35]]
df = pd.DataFrame(data, columns=['Name', 'Age'])
# 从字典创建 DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
# 从 NumPy 数组创建 DataFrame
import numpy as np
data = np.array([['Alice', 25], ['Bob', 30], ['Charlie', 35]])
df = pd.DataFrame(data, columns=['Name', 'Age'])
```
2. 从文件读取数据创建:
```python
import pandas as pd
# 从 CSV 文件读取数据创建 DataFrame
df = pd.read_csv('data.csv')
# 从 Excel 文件读取数据创建 DataFrame
df = pd.read_excel('data.xlsx')
```
DataFrame 提供了丰富的方法和属性,可以进行数据的筛选、切片、聚合、合并等操作,以及统计分析和可视化。
相关推荐
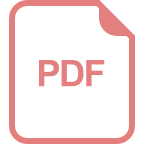
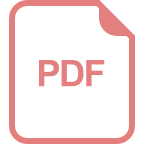












