Python中字符串比较
时间: 2024-08-16 13:03:52 浏览: 77
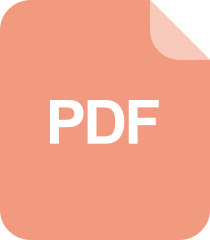
Python中文字符串截取问题

在Python中,字符串是比较常见的数据类型,可以使用多种方式进行比较。以下是几种常用的字符串比较方式:
1. 直接相等比较 (`==`): 比较两个字符串是否完全相同。例如:
```python
str1 = "hello"
str2 = "hello"
if str1 == str2:
print("Strings are equal")
```
2. 长度比较 (`len()`): 可以比较两个字符串的长度,而不考虑内容。如:
```python
str1 = "short"
str2 = "longer"
if len(str1) < len(str2):
print(f"{str1} is shorter than {str2}")
```
3. 按字典序排序 (`sorted()`, `min()`, `max()`): 可以对字符串进行排序。默认按字母顺序排列,对于Unicode字符也有对应的排序规则。
```python
words = ["cat", "dog", "apple"]
longest_word = max(words, key=len)
```
4. 利用`casefold()`方法进行大小写不敏感比较: 对于某些特定应用场景,如搜索、拼写检查等,可能需要忽略大小写差异。
```python
string1 = "Hello"
string2 = "hello"
if string1.casefold() == string2.casefold():
print("Both strings are the same ignoring case")
```
5. 正则表达式匹配 (`re`模块): 更复杂的情况可以用正则表达式进行模式匹配。
阅读全文
相关推荐
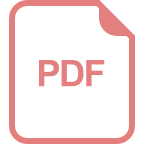
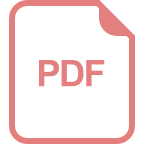
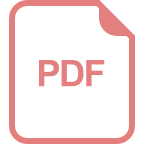
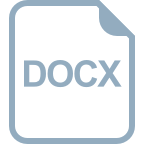
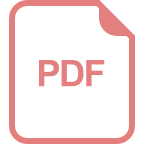
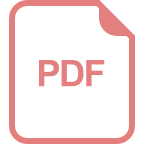
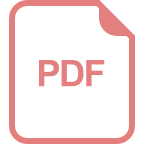
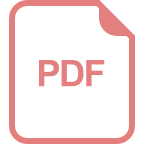
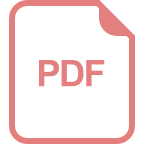
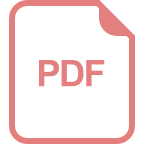
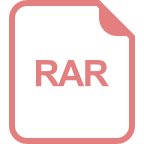
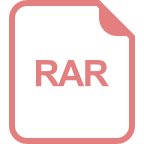
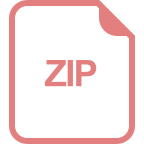
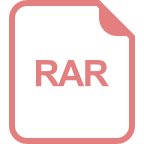