如何对二值化描述子的匹配点对进行滤除
时间: 2024-02-22 11:59:09 浏览: 18
对于二值化的特征描述子,可以使用以下方法对匹配点对进行滤除:
1. 使用汉明距离作为匹配度量,计算所有匹配点对的汉明距离。
2. 将所有汉明距离按照从小到大的顺序排序。
3. 如果最小距离小于一个阈值,则将其作为正确匹配点,将其从匹配点对中去除。
4. 从剩余的匹配点对中,选择一个匹配度最高的点对作为正确匹配点,将其从匹配点对中去除,并将其对应的两个点从点云中去除。
5. 重复步骤4,直到没有匹配点对剩余为止。
需要注意的是,在使用二值化的描述子进行匹配的过程中,误匹配的情况比较常见,因此需要设置较小的误差阈值,并进行适当的滤除。同时,在进行滤除时,要注意不要将正确匹配的点对滤除掉。
以下是一个简单的示例代码,展示了如何使用汉明距离对二值化的描述子匹配点对进行滤除:
```cpp
// 定义描述子类型及相关变量
typedef pcl::SHOT352 Descriptor;
typedef pcl::PointCloud<Descriptor> DescriptorCloud;
DescriptorCloud::Ptr descriptors_src(new DescriptorCloud);
DescriptorCloud::Ptr descriptors_tgt(new DescriptorCloud);
// 计算匹配点对及其汉明距离
pcl::Correspondences all_correspondences;
pcl::registration::CorrespondenceEstimation<Descriptor, Descriptor> est;
est.setInputSource(descriptors_src);
est.setInputTarget(descriptors_tgt);
est.determineCorrespondences(all_correspondences);
// 将汉明距离按照从小到大的顺序排序
std::sort(all_correspondences.begin(), all_correspondences.end(),
[](const pcl::Correspondence& a, const pcl::Correspondence& b) {
return a.distance < b.distance; });
// 设置误差阈值,将小于阈值的匹配点对作为正确匹配点
const float kErrorThreshold = 20.0f;
pcl::Correspondences correspondences;
for (const auto& correspondence : all_correspondences) {
if (correspondence.distance > kErrorThreshold) {
correspondences.push_back(correspondence);
}
}
// 逐步滤除误匹配点
while (true) {
if (correspondences.empty()) {
break;
}
const auto& correspondence = correspondences.front();
correspondences.erase(correspondences.begin());
// 检查该匹配点对是否已经被删除
if (correspondence.index_query >= static_cast<int>(cloud_src->size()) ||
correspondence.index_match >= static_cast<int>(cloud_tgt->size())) {
continue;
}
// 将正确匹配的点对从点云中删除
cloud_src->erase(cloud_src->begin() + correspondence.index_query);
cloud_tgt->erase(cloud_tgt->begin() + correspondence.index_match);
// 重新计算匹配点对
est.setInputSource(descriptors_src);
est.setInputTarget(descriptors_tgt);
correspondences.clear();
est.determineCorrespondences(correspondences);
}
```
在以上代码中,我们首先计算了匹配点对及其汉明距离,并将汉明距离按照从小到大的顺序排序。然后,我们将距离小于一个阈值的匹配点对作为正确匹配点,并将其从匹配点对中去除。接着,我们逐步滤除匹配度最高的点对,直到没有匹配点对剩余为止。在滤除点对的过程中,我们使用了`erase`函数从点云中删除了对应的点。
相关推荐
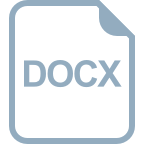
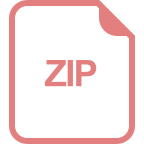
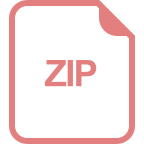














