给我一个表单校验的实例,要求在vue3,ts下,对a-input表单做出校验,当点击a-button组件时,若a-input组件为空,则弹出“请输入ID”
时间: 2024-06-01 10:10:50 浏览: 121
<template>
<div>
<a-input v-model="id" placeholder="请输入ID" />
<a-button type="primary" @click="handleSubmit">提交</a-button>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
data() {
return {
id: '',
};
},
methods: {
handleSubmit() {
if (!this.id) {
alert('请输入ID');
return;
}
// 其他校验逻辑
// 提交数据
console.log('提交数据:', this.id);
},
},
});
</script>
阅读全文
相关推荐
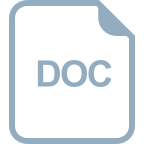
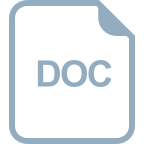
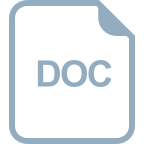
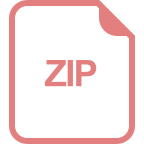
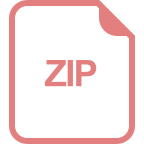
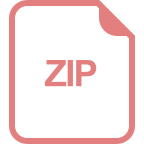
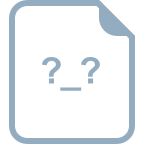
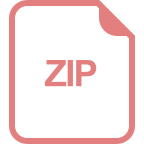
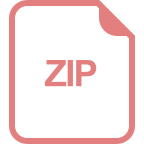
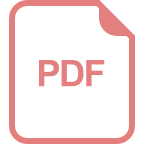
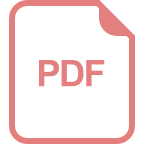
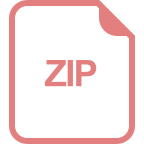
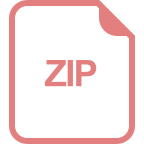
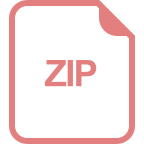
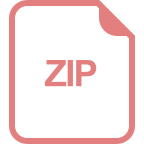
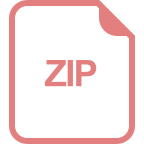
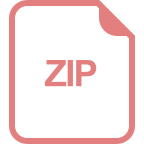
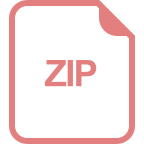