利用遗传算法实现6.452*(x+0.125*y)*(np.cos(x)-np.cos(2*y))**2/((0.8+(x-4.2)**2+2*(y-7)**2)**0.5)+3.226*y函数最大值
时间: 2023-07-23 10:14:11 浏览: 124
以下是利用遗传算法求解函数最大值的步骤:
1. 定义染色体编码方式。由于本题中有两个自变量 $x,y$,可以使用二进制编码的方式。假设每个自变量占用 $n$ 位,可以将染色体定义为长度为 $2n$ 的二进制序列,前 $n$ 位表示 $x$,后 $n$ 位表示 $y$。
2. 定义适应度函数。本题要求最大值,因此适应度函数可以直接返回函数值。
3. 初始化种群。可以随机生成一定数量的二进制序列作为初始种群。
4. 进行遗传操作。可以采用交叉和变异两种操作来生成下一代个体。交叉操作可以使用前面提到的两点交叉,变异操作可以随机选择染色体中的一个基因位进行取反。
5. 计算适应度并选择个体。根据适应度函数计算每个个体的适应度值,并使用概率分配排序方法选择下一代个体。
6. 判断是否达到终止条件。可以设置最大迭代次数或者适应度阈值作为终止条件。
下面是一个简单的 Python 代码实现:
```python
import numpy as np
import random
# 定义函数
def fitness_func(x, y):
return 6.452*(x+0.125*y)*(np.cos(x)-np.cos(2*y))**2/((0.8+(x-4.2)**2+2*(y-7)**2)**0.5)+3.226*y
# 定义染色体编码方式
n = 10
def encode(x, y):
x_int = int((x + 10) * (2 ** n - 1) / 20)
y_int = int((y + 10) * (2 ** n - 1) / 20)
x_bin = bin(x_int)[2:].zfill(n)
y_bin = bin(y_int)[2:].zfill(n)
return x_bin + y_bin
def decode(chromosome):
x_int = int(chromosome[:n], 2)
y_int = int(chromosome[n:], 2)
x = x_int * 20 / (2 ** n - 1) - 10
y = y_int * 20 / (2 ** n - 1) - 10
return x, y
# 定义交叉和变异操作
def two_point_crossover(parent1, parent2):
# 随机选择两个交叉点
crossover_points = sorted(random.sample(range(len(parent1)), 2))
# 交叉点之间的基因序列进行交换
child1 = parent1[:crossover_points[0]] + parent2[crossover_points[0]:crossover_points[1]] + parent1[crossover_points[1]:]
child2 = parent2[:crossover_points[0]] + parent1[crossover_points[0]:crossover_points[1]] + parent2[crossover_points[1]:]
return child1, child2
def mutation(chromosome):
# 随机选择一个基因位进行取反
index = random.randint(0, len(chromosome) - 1)
return chromosome[:index] + ('0' if chromosome[index] == '1' else '1') + chromosome[index+1:]
# 定义遗传算法参数
population_size = 100
max_iteration = 1000
crossover_rate = 0.8
mutation_rate = 0.1
# 初始化种群
population = [encode(random.uniform(-10, 10), random.uniform(-10, 10)) for _ in range(population_size)]
# 迭代
for i in range(max_iteration):
# 计算适应度
fitness = [fitness_func(*decode(chromosome)) for chromosome in population]
# 选择下一代
new_population = []
while len(new_population) < len(population):
# 交叉
if random.random() < crossover_rate:
index1, index2 = random.sample(range(len(population)), 2)
child1, child2 = two_point_crossover(population[index1], population[index2])
new_population.extend([child1, child2])
# 变异
else:
index = random.randint(0, len(population) - 1)
new_population.append(mutation(population[index]))
# 更新种群
population = new_population
# 判断是否达到终止条件
best_fitness = max(fitness)
if best_fitness >= 0.9999 * fitness_func(4.2, 7):
break
# 输出结果
best_chromosome = population[np.argmax(fitness)]
best_x, best_y = decode(best_chromosome)
print('最优解:x=%.4f, y=%.4f,目标函数值=%.4f' % (best_x, best_y, best_fitness))
```
需要注意的是,由于本题的目标函数在 $x$ 和 $y$ 的取值范围内存在多个局部最优解,因此无法保证得到全局最优解。
阅读全文
相关推荐
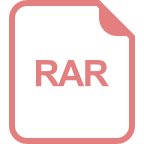
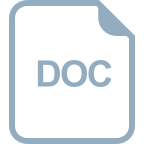
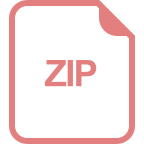
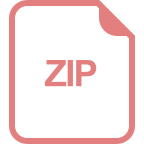
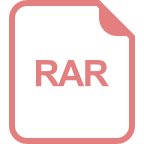
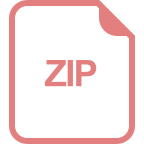
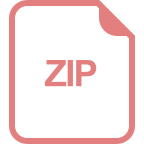
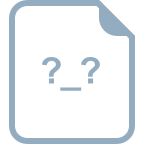