img_numpy = np.transpose(img.mul(0.5).add(0.5).mul(255).cpu().numpy(), (0, 2, 3, 1)).astype(np.uint8) for mask, out_img, imgpath, pad in zip(preds, img_numpy, path, pad_scope): src_img = cv2.imdecode(np.fromfile(imgpath, dtype=np.uint8), -1)
时间: 2024-03-20 07:43:59 浏览: 11
这段代码主要完成了以下几个功能:
1. 将 PyTorch 的 tensor 类型的图像数据 img 转换为 numpy 数组类型的图像数据 img_numpy,并将像素值从 [-1,1] 转换为 [0,255] 的 uint8 类型。具体实现过程为:
```
img_numpy = np.transpose(img.mul(0.5).add(0.5).mul(255).cpu().numpy(), (0, 2, 3, 1)).astype(np.uint8)
```
2. 使用 zip 函数和 for 循环对四个列表进行迭代,分别是 preds、img_numpy、path 和 pad_scope。在每次迭代中,分别取出这四个列表中对应位置的元素,分别命名为 mask、out_img、imgpath 和 pad。
3. 使用 OpenCV 的 imdecode 函数读取 imgpath 指定的图片文件,并将其解码成 numpy 数组类型的图像数据 src_img。
这段代码的作用可能是将模型输出的 mask 与原始图像进行融合,生成带有 mask 的特效图像。
相关问题
from bm3d import bm3d_rgb from experiment_funcs1 import get_experiment_noise, get_psnr, get_cropped_psnr from PIL import Image import argparse import os import torch import numpy as np from torchvision.utils import save_image def main(): imagename = './test_image1/(1271).jpg' save_dir = 'test_result' save_path = 'noise' y = np.array(Image.open(imagename)) / 255 noise_type = 'g3' noise_var = 0.02 seed = 0 noise, psd, kernel = get_experiment_noise(noise_type, noise_var, seed, y.shape) z = np.atleast_3d(y) + np.atleast_3d(noise) y_est = bm3d_rgb(z, psd) psnr = get_psnr(y, y_est) print("PSNR:", psnr) y_est = np.minimum(np.maximum(y_est, 0), 1) z_rang = np.minimum(np.maximum(z, 0), 1) z_rang = torch.from_numpy(np.transpose(z_rang, (2, 0, 1))).float() y_est = torch.from_numpy(np.transpose(y_est, (2, 0, 1))).float() denoise_img_path = os.path.join(save_dir, 'denoised.jpg') save_image(y_est, denoise_img_path) noise_img_path = os.path.join(save_path, 'noise.jpg') save_image(z_rang, noise_img_path) if __name__ == '__main__': main()改为对灰度图处理
可以将代码中的`bm3d_rgb`函数改为`bm3d`函数,同时将读入图像的方式改为读入灰度图像即可。具体代码如下:
```
from bm3d import bm3d
from experiment_funcs1 import get_experiment_noise, get_psnr, get_cropped_psnr
from PIL import Image
import argparse
import os
import torch
import numpy as np
from torchvision.utils import save_image
def main():
imagename = './test_image1/(1271).jpg'
save_dir = 'test_result'
save_path = 'noise'
y = np.array(Image.open(imagename).convert('L')) / 255
noise_type = 'g3'
noise_var = 0.02
seed = 0
noise, psd, kernel = get_experiment_noise(noise_type, noise_var, seed, y.shape)
z = y + noise
y_est = bm3d(z, psd)
psnr = get_psnr(y, y_est)
print("PSNR:", psnr)
y_est = np.minimum(np.maximum(y_est, 0), 1)
z_rang = np.minimum(np.maximum(z, 0), 1)
z_rang = torch.from_numpy(z_rang).unsqueeze(0).float()
y_est = torch.from_numpy(y_est).unsqueeze(0).float()
denoise_img_path = os.path.join(save_dir, 'denoised.jpg')
save_image(y_est, denoise_img_path)
noise_img_path = os.path.join(save_path, 'noise.jpg')
save_image(z_rang, noise_img_path)
if __name__ == '__main__':
main()
```
from skimage.segmentation import slic, mark_boundaries import torchvision.transforms as transforms import numpy as np from PIL import Image import matplotlib.pyplot as plt import cv2 # 加载图像 image = Image.open('img.png') # 转换为 PyTorch 张量 transform = transforms.ToTensor() img_tensor = transform(image).unsqueeze(0) # 将 PyTorch 张量转换为 Numpy 数组 img_np = img_tensor.numpy().transpose(0, 2, 3, 1)[0] # 使用 SLIC 算法生成超像素标记图 segments = slic(img_np, n_segments=100, compactness=10) # 可视化超像素标记图 segment_img = mark_boundaries(img_np, segments) # 将 Numpy 数组转换为 PIL 图像 segment_img = Image.fromarray((segment_img * 255).astype(np.uint8)) # 保存超像素标记图 segment_img.save('segments.jpg') n_segments = np.max(segments) + 1 # 初始化超像素块的区域 segment_regions = np.zeros((n_segments, img_np.shape[0], img_np.shape[1])) # 遍历每个超像素块 for i in range(n_segments): # 获取当前超像素块的掩码 mask = (segments == i) # 将当前超像素块的掩码赋值给超像素块的区域 segment_regions[i][mask] = 1 # 保存超像素块的区域 np.save('segment_regions.npy', segment_regions) # 加载超像素块的区域 segment_regions = np.load('segment_regions.npy') # 取出第一个超像素块的区域 segment_region = segment_regions[0] segment_region = (segment_region * 255).astype(np.uint8) # 显示超像素块的区域 plt.imshow(segment_region, cmap='gray') plt.show(),将上述代码中,# 取出第一个超像素块的区域 segment_region = segment_regions[0]改为取出全部超像素块的区域
如果你想获取所有超像素块的区域,可以将`segment_regions[0]`改成`segment_regions`即可,代码如下:
```python
from skimage.segmentation import slic, mark_boundaries
import torchvision.transforms as transforms
import numpy as np
from PIL import Image
import matplotlib.pyplot as plt
import cv2
# 加载图像
image = Image.open('img.png')
# 转换为 PyTorch 张量
transform = transforms.ToTensor()
img_tensor = transform(image).unsqueeze(0)
# 将 PyTorch 张量转换为 Numpy 数组
img_np = img_tensor.numpy().transpose(0, 2, 3, 1)[0]
# 使用 SLIC 算法生成超像素标记图
segments = slic(img_np, n_segments=100, compactness=10)
# 可视化超像素标记图
segment_img = mark_boundaries(img_np, segments)
# 将 Numpy 数组转换为 PIL 图像
segment_img = Image.fromarray((segment_img * 255).astype(np.uint8))
# 保存超像素标记图
segment_img.save('segments.jpg')
n_segments = np.max(segments) + 1
# 初始化超像素块的区域
segment_regions = np.zeros((n_segments, img_np.shape[0], img_np.shape[1]))
# 遍历每个超像素块
for i in range(n_segments):
# 获取当前超像素块的掩码
mask = (segments == i)
# 将当前超像素块的掩码赋值给超像素块的区域
segment_regions[i][mask] = 1
# 保存超像素块的区域
np.save('segment_regions.npy', segment_regions)
# 加载超像素块的区域
segment_regions = np.load('segment_regions.npy')
# 取出所有超像素块的区域
segment_regions = (segment_regions * 255).astype(np.uint8)
# 显示超像素块的区域
for i in range(n_segments):
plt.imshow(segment_regions[i], cmap='gray')
plt.show()
```
这个代码会显示出所有超像素块的区域。注意,如果超像素块数量太多,这个过程可能会很慢。
相关推荐









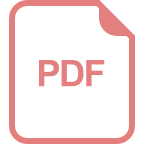
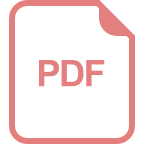